1.1 1.2 1.3 1.3.1 1.4 1.4.1 1.4.2 1.5 1.5.1 1.5.2 2.1 2.2 2.3 2.4 2.5 2.6 2.7 2
1.1 1.2 1.3 1.3.1 1.4 1.4.1 1.4.2 1.5 1.5.1 1.5.2 2.1 2.2 2.3 2.4 2.5 2.6 2.7 2.8 2.9 2.10 Table of Contents Introduction Why Map? Getting Started Usage Mappings via XML via Annotations via API Configuration Advanced Configuration Global Configuration Dozer mapping concepts Mapping Classes Basic Property Mapping Inheritance Mapping Context Based Mapping One-Way Mapping Copying By Object Reference Deep Property Mapping Indexed Property Mapping Excluding Fields Assembler Pattern How do i map a type of…? 1 3.1 3.2 3.3 3.4 3.5 4.1 4.2 4.3 4.4 4.5 5.1 5.2 5.3 5.4 5.5 6.1 6.2 Enums String to Date Collections and Arrays Map Backed Property Mapping Proxy Objects What if i need to customise the mapping? Custom Converters Custom Bean Factories Custom Create Methods Custom get() set() Methods Expression Language Extra Logging Event Listening Statistics JMX Integration Metadata Query Interface 3rd Party Spring Integration JAXB and XMLBeans Other 2 7.1 7.2 8.1 8.2 9.1 9.2 10.1 10.2 10.2.1 10.2.2 FAQ Examples Migration v5 to v6 v6.0.0 to v6.1.0 Schema schema/bean-mapping.xsd[Dozer Mapping XSD] dozer-user-guide.pdf[User’s Guide PDF] Eclipse Plugin Installation Usage via XML via Editor 3 Dozer What is Dozer? Dozer is a Java Bean to Java Bean mapper that recursively copies data from one object to another. Typically, these Java Beans will be of different complex types. Dozer supports simple property mapping, complex type mapping, bi-directional mapping, implicit-explicit mapping, as well as recursive mapping. This includes mapping collection attributes that also need mmapping at the element level. Please read the about page for detailed information on Dozer. Introduction 4 Why Map? A mapping framework is useful in a layered architecture where you are creating layers of abstraction by encapsulating changes to particular data objects vs. propagating these objects to other layers (i.e. external service data objects, domain objects, data transfer objects, internal service data objects). A mapping framework is ideal for using within Mapper type classes that are responsible for mapping data from one data object to another. For distributed systems, a side effect is the passing of domain objects between different systems. Typically, you won’t want internal domain objects exposed externally and won’t allow for external domain objects to bleed into your system. Mapping between data objects has been traditionally addressed by hand coding value object assemblers (or converters) that copy data between the objects. Most programmers will develop some sort of custom mapping framework and spend countless hours and thousands of lines of code mapping to and from their different data object. A generic mapping framework solves these problems. Dozer is an open source mapping framework that is robust, generic, flexible, reusable, and configurable. Data object mapping is an important part of layered service oriented architectures. Pick and choose the layers you use mapping carefully. Do not go overboard as there is maintenance and performance costs associated with mapping data objects between layers. Parallel Object Hierarchies There could be different reasons of why application should support parallel object hierarhchies. To name a few: Integration with External Code Serialization Requirements Framework Integration Separation of Architectural Layers Why Map? 5 In some cases it is efficient to guard your code base from frequently changing object hierarchy, which you do not control directly. In this case Dozer serves as a bridge between application and external objects. As mapping is performed in reflective manner not all changes break your API. For example if object changes from Number to String the code will keep working as this is resolved automatically. Some frameworks impose Serialization constraints, which does not allow sending any Java objects through the wire. One of the popular examples is Google Web Toolkit (GWT) framework, which restricts developer to sending only objects compiled to JavaScript and marked as Serializable. Dozer helps to convert Rich Domain Model to Presentation Model, which satisfies GWT serialization requirements. Dozer integrates nicely with frameworks such as Apache XmlBeans and JAXB implementations. With help of provided factory classes, conversion between domain model and Xml objects is defined in the same way as plain object to object mappings. In complex enterprise application it is often valuable to separate design to several architectural layers. Each of them would reside on its own abstraction level. A typical simplified example would be presentation, domain and persistence layers. Each of this layers could have own set of Java Beans representing data relevant for this layer. It is not necessary that all data will travel to the upper levels of architecture. For example the same domain object could have different mappings dependant of the presentation layer requirements. Why Map? 6 Getting Started Downloading the Distribution If you are using Apache Maven, simply copy-paste this dependency to your project. <dependency> <groupId>net.sf.dozer</groupId> <artifactId>dozer</artifactId> <version>6.1.0</version> </dependency> 1st Mapping For your first mapping, lets assume that the two data objects share all common attribute names. Mapper mapper = DozerBeanMapperBuilder.createDefault(); DestinationObject destObject = mapper.map(sourceObject, DestinationObject.class); After performing the Dozer mapping, the result will be a new instance of the destination object that contains values for all fields that have the same field name as the source object. If any of the mapped attributes are of different data types, the Dozer mapping engine will automatically perform data type conversion. At this point you have completed your first Dozer mapping. Later sections will go over how to specify custom mappings via custom xml files. IMPORTANT: For real-world applications it is NOT recommended to create a new instance of the Mapper each time you map objects but reuse created instance instead. Specifying Custom Mappings via XML If the two different types of data objects that you are mapping contain any fields that don’t share a common property name, you will need to add a class mapping entry to your custom mapping xml file. These mappings xml files are used at runtime by the Getting Started 7 Dozer mapping engine. Dozer automatically performs any type conversion when copying the source field data to the destination field. The Dozer mapping engine is bi-directional, so if you wanted to map the destination object to the source object, you do not need to add another class mapping to the xml file. IMPORTANT: Fields that are of the same name do not need to be specified in the mapping xml file. Dozer automatically maps all fields with the same property name from the source object into the destination object. i.e.: <mapping> <class-a>yourpackage.yourSourceClassName</class-a> <class-b>yourpackage.yourDestinationClassName</class-b> <field> <a>yourSourceFieldName</a> <b>yourDestinationFieldName</b> </field> </mapping> The complete Dozer mapping xml file would look like the following. The Custom Mappings section contains more information on mapping options that are available to you for more complex use cases. <?xml version="1.0" encoding="UTF-8"?> <mappings xmlns="http://dozermapper.github.io/schema/bean-mapping" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://dozermapper.github.io/schema/bean-mapping http:// dozermapper.github.io/schema/bean-mapping.xsd"> <configuration> <stop-on-errors>true</stop-on-errors> <date-format>MM/dd/yyyy HH:mm</date-format> <wildcard>true</wildcard> </configuration> <mapping> <class-a>yourpackage.yourSourceClassName</class-a> <class-b>yourpackage.yourDestinationClassName</class-b> <field> <A>yourSourceFieldName</A> <B>yourDestinationFieldName</B> </field> </mapping> Getting Started 8 </mappings> Dozer and Dependency Injection Frameworks Dozer is not dependant of any existing Dependency Injection framework (DI). However the general aim is to support the most typical use cases with ready-to-use wrappers. Check Spring Integration manual for option of initializing Dozer in context of Spring DI framework. Getting Started 9 Usage Dozer Bean Mapper Before we go over setting up custom xml bean mappings, let us look at a simple example of using Dozer. The Dozer mapping implementation has a method called map which takes a source object and either a destination object or destination object class type. After mapping the two objects it then returns the destination object with all of its mapped fields. Mapper mapper = DozerBeanMapperBuilder.createDefault(); DestinationObject destObject = mapper.map(sourceObject, DestinationObject.class); Or… DestinationObject destObject = new DestinationObject(); mapper.map(sourceObject, destObject); Dozer operates in two general modes: implicit and explicit. Implicit mode is activated by default and tries to resolve mappings for you. It uses simple assumptions that if two objects are passed for mapping then bean properties with the same names should be mapped. If there are additional mappings needed, which can not be derived by the naming you should add those either via XML, annotations or API. Explicit mode assumes that no mappings should be performed or "guessed" until you have specified those specifically. The amount of coding is higher in explicit mode, but sometimes you would like to have full control on what is going on during the mappings process and this approach is also used in many of the productive applications. Implicit/Explicit mapping switch is called "wildcard" in Dozer. Injecting Custom Mapping Files Usage 10 The Dozer mapping xml file(s) define any custom mappings that can’t be automatically performed by the Dozer mapping engine. Any custom Dozer mapping files need to be injected into the Mapper implementation via DozerBeanMapperBuilder#withMappingFiles(..) method. Preferably, you will be using an IOC framework such as Spring for these Dozer injection requirements. Alternatively, the injection of mapping files can be done programmatically. Below is a programmatic approach to creating a bean mapper. Note that this is NOT the recommended way to retrieve the bean mapper. Each new instance needs to be initialized and this consumes time as well as resources. If you are using the mapper this way just wrap it using the uploads/s1/ dozer-user-guide.pdf
Documents similaires
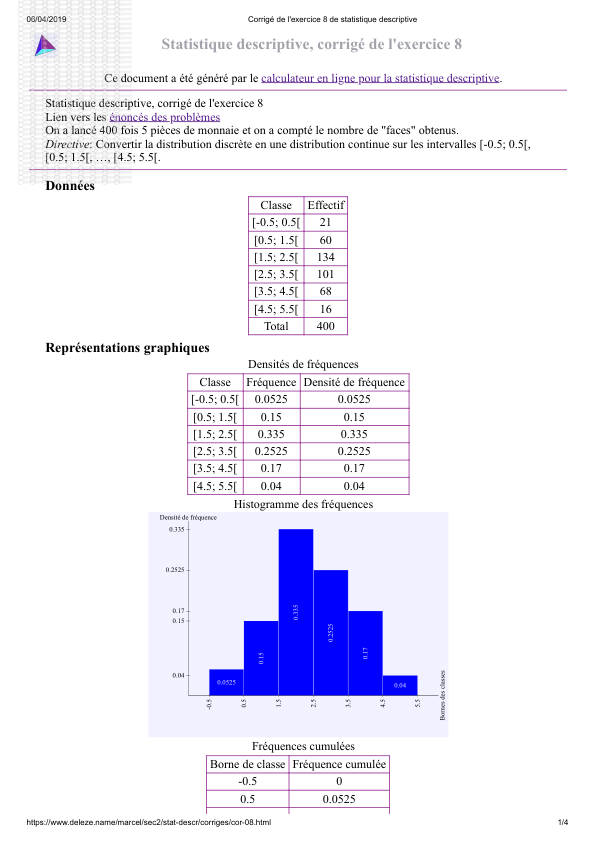
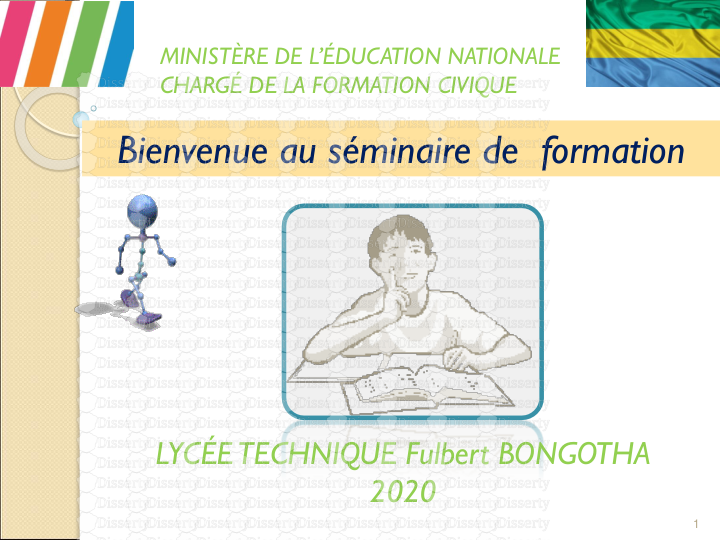
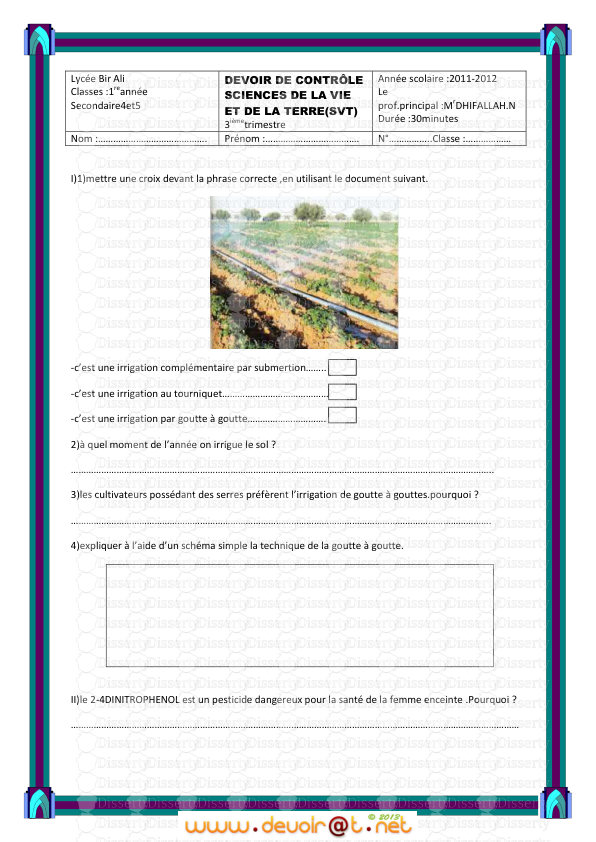
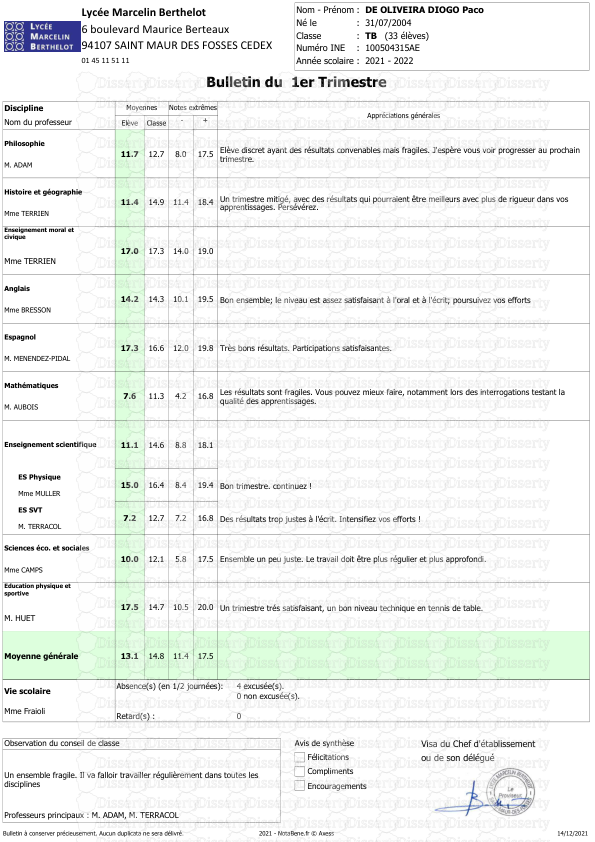
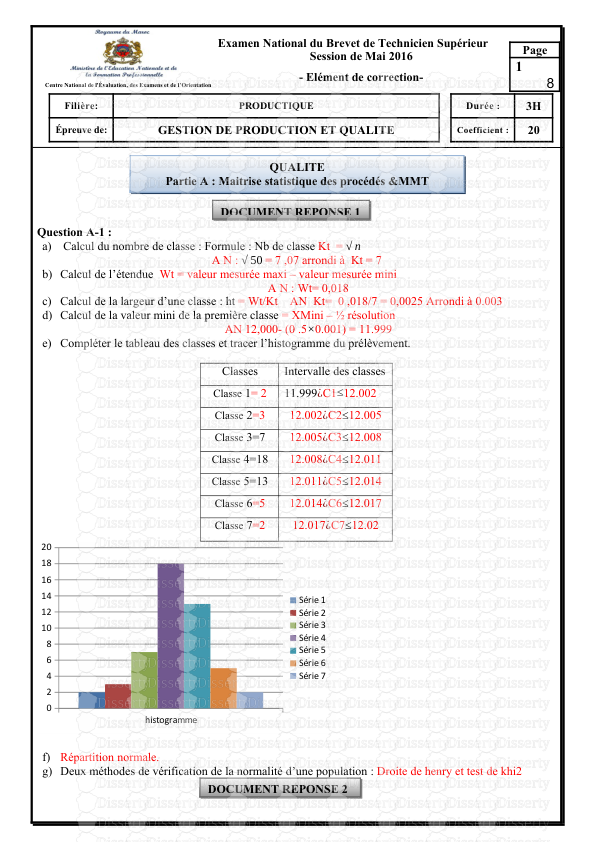
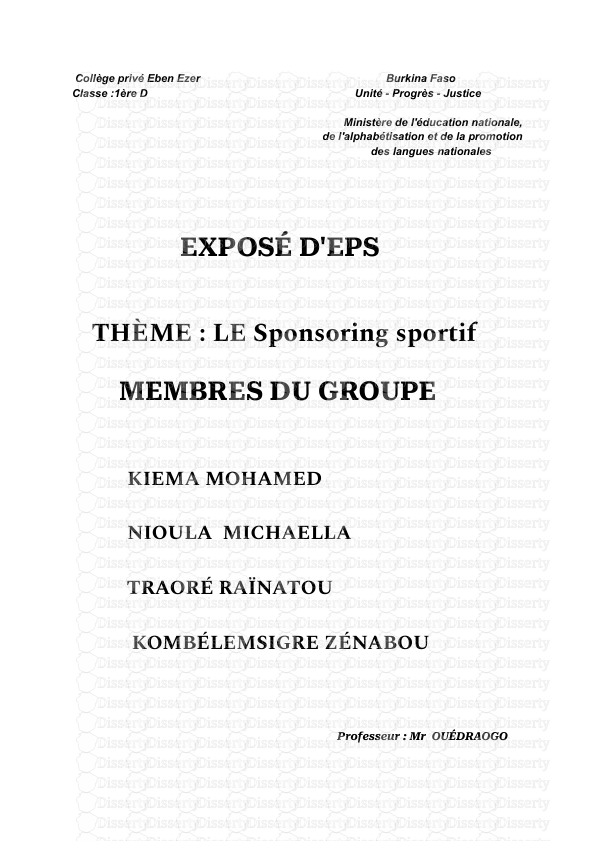
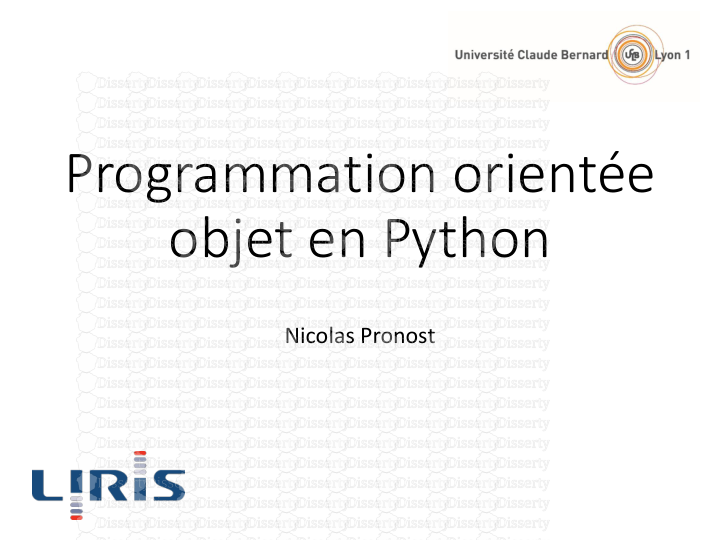
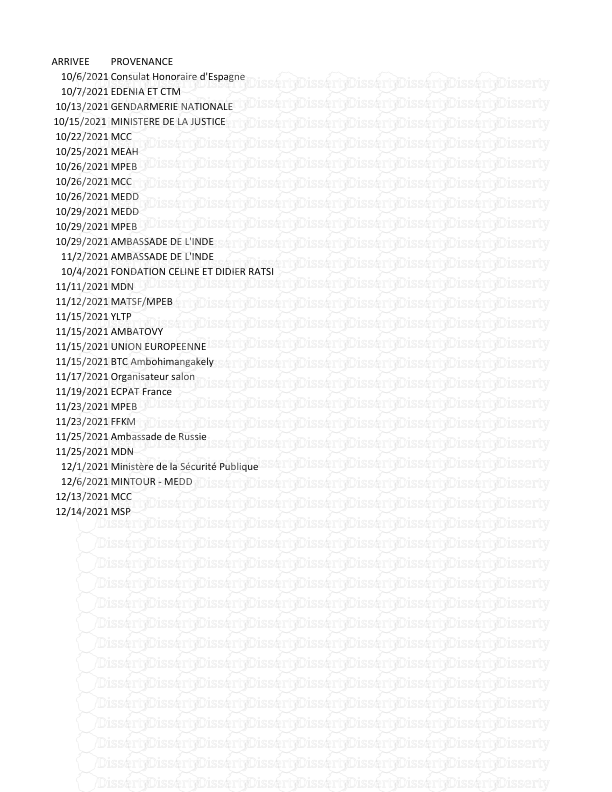
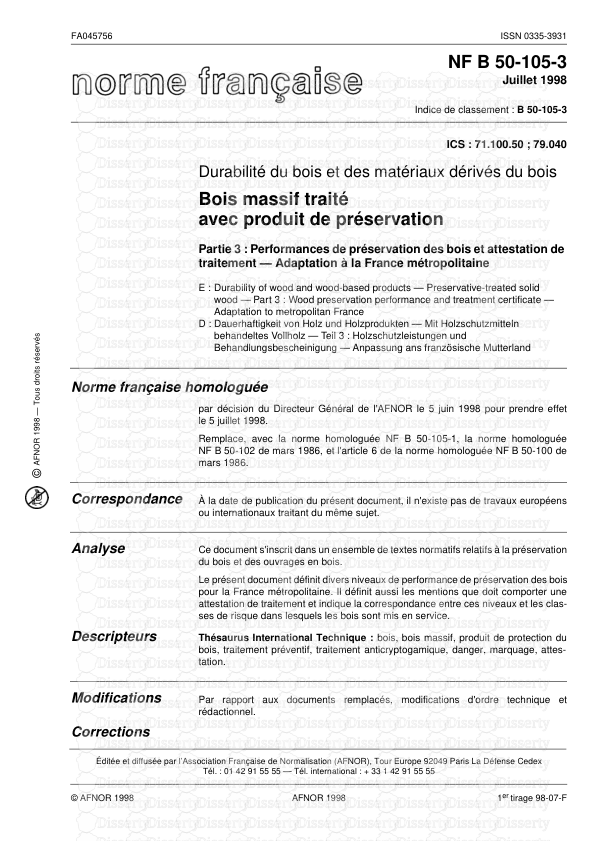
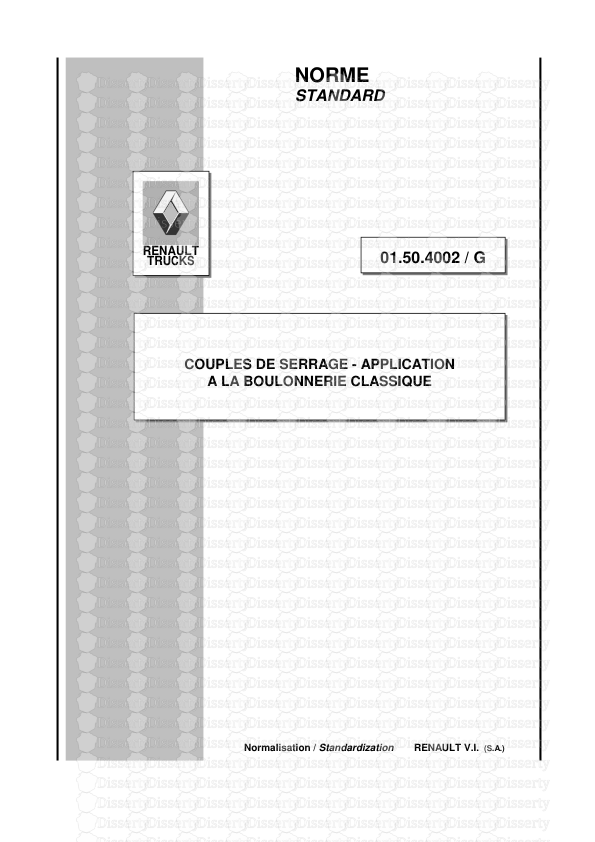
-
38
-
0
-
0
Licence et utilisation
Gratuit pour un usage personnel Attribution requise- Détails
- Publié le Aoû 18, 2021
- Catégorie Administration
- Langue French
- Taille du fichier 1.1651MB