Master 2 BBSG POO, langage Java Laurent Tichit 1. Des exercices simples pour co
Master 2 BBSG POO, langage Java Laurent Tichit 1. Des exercices simples pour commencer 1.1. L’incontournable « Print "Hello" » public class Bonjour { public static void main(String[] args) { System.out.println("Bonjour"); } } Sans commentaire... 1.2. Les arguments de la ligne de commande public class Calcul { public static void main(String[] args) { if (args.length < 3) { System.out.println("Emploi: java Calcul <nombre> <opérateur> <nombre>"); return; } double x = Double.parseDouble(args[0]); double y = Double.parseDouble(args[2]); double z = 0; switch (args[1].charAt(0)) { case '+': z = x + y; break; case '-': z = x - y; break; case '*': z = x * y; break; case '/': z = x / y; break; } System.out.println(x + " " + args[1] + " " + y + " = " + z); } } 1.3. Jeux avec les chaînes public class JeuxDeChaines { public static void main(String[] args) { int i = Integer.valueOf(args[0]); String s1 = args[1]; String s2 = args[2]; /* A */ String s = String.valueOf(i); System.out.println("résultat: \"" + s + "\""); /* B */ i = Integer.parseInt(s) + 1; System.out.println("résultat: " + i); /* C */ double x = Double.parseDouble(s) + 1; System.out.println("résultat: " + x); /* D */ System.out.println("Chaine lue '" + s1 + "'"); s = s1.trim(); System.out.println("Chaine nette '" + s + "'"); s = s.toUpperCase(); System.out.println("Chaine capitalisée '" + s + "'"); /* E */ System.out.print("elles commencent par la meme lettre? "); if (s1.length() > 0 && s2.length() > 0 && s1.charAt(0) == s2.charAt(0)) System.out.println("oui"); else System.out.println("non"); /* F */ System.out.println("s1 == s2 : " + (s1 == s2)); System.out.println("s1.equals(s2) : " + s1.equals(s2)); System.out.println("s1.compareTo(s2) : " + s1.compareTo(s2)); System.out.println("s1.compareToIgnoreCase(s2) : " + s1.compareToIgnoreCase(s2)); /* G */ System.out.println("'" + s1 + "' commence par '" + s2 + "' : " + s1.startsWith(s2)); System.out.println("'" + s1 + "' finit par '" + s2 + "' : " + s1.endsWith(s2)); System.out.println("'" + s1 + "' contient '" + s2 + "' : " + s1.contains(s2)); System.out.println("'" + s1 + "' contient '" + s2 + "' : " + (s1.indexOf(s2) >= 0)); /* H */ i = s1.indexOf(s2); if (i >= 0) s1 = s1.substring(0, i) + s1.substring(i + s2.length()); System.out.println("s1 sans s2 : '" + s1 + "'"); /* I */ if ( ! (s1.equals("bonjour") && s2.equals("bonjour"))) System.out.println("Suivez les instructions!"); else { System.out.println("s1.equals(s2) est " + s1.equals(s2)); System.out.println("\ns1 == s2 est " + (s1 == s2)); System.out.println("s1 == \"bonjour\" est " + (s1 == "bonjour")); System.out.println("\non fait s1 = s1.intern()"); s1 = s1.intern(); System.out.println("s1 == s2 est encore " + (s1 == s2)); System.out.println("mais s1 == \"bonjour\" est deja " + (s1 == "bonjour")); System.out.println("\non fait s2 = s2.intern()"); s2 = s2.intern(); System.out.println("enfin, même s1 == s2 est " + (s1 == s2)); } } } 1.4. Random public class RandomUn { public static void main(String[] args) { int nombre = 100000; double somme = 0; double sommeCarres = 0; for (int i = 0; i < nombre; i++) { double x = Math.random(); somme += x; sommeCarres += x * x; } double moyenne = somme / nombre; double ecart = Math.sqrt(sommeCarres / nombre - moyenne * moyenne); System.out.println("moyenne " + moyenne + ", ecart-type " + ecart); } } Pas de commentaire non plus. La documentation officielle présente la fonction Math.random() comme une manière simple d’utiliser une instance de la classe plus complète java.util.Random mais, à défaut d’information plus précise, il faut penser que les algorithmes utilisés par Math.random() et unObjetRandom.nextDouble() sont les mêmes. 1.5. Calcul de la factorielle import java.math.BigInteger; public class Factorielle { /* question A */ static long factorielle1(int n) { long result = 1; while (n > 0) result *= (n--); return result; } /* question B */ static BigInteger factorielle2(int n) { BigInteger result = BigInteger.ONE; while (n > 0) result = result.multiply(BigInteger.valueOf(n--)); return result; } public static void main(String[] args) { System.out.println("Test 1"); long factPrec1 = 1; for (int n = 1; n < 25; n++) { long fact = factorielle1(n); System.out.println(" " + n + " ! = " + fact + " (rapport: " + fact / factPrec1 + ")"); factPrec1 = fact; } System.out.println("Test 2"); BigInteger factPrec2 = BigInteger.ONE; for (int n = 1; n < 100; n++) { BigInteger fact = factorielle2(n); System.out.println(" " + n + " ! = " + fact + " (rapport: " + fact.divide(factPrec2) + ")"); factPrec2 = fact; } } } La transformation de factorielle1 en factorielle2 se passe de commentaires. Affichage obtenu : TTest 1 1 ! = 1 (rapport: 1) 2 ! = 2 (rapport: 2) ... 19 ! = 121645100408832000 (rapport: 19) 20 ! = 2432902008176640000 (rapport: 20) 21 ! = -4249290049419214848 (rapport: -1) 22 ! = -1250660718674968576 (rapport: 0) ... Test 2 1 ! = 1 (rapport: 1) 2 ! = 2 (rapport: 2) ... 98 ! = 942689044888324774562618574305724247380969376407895166349423877729470707002 322379888297615920772911982360585058860846042941264756736000000000000000000 0000 (rapport: 98) 99 ! = 933262154439441526816992388562667004907159682643816214685929638952175999932 299156089414639761565182862536979208272237582511852109168640000000000000000 000000 (rapport: 99) 1.6. Quel jour sommes-nous ? quelle heure est-il ? import java.text.*; import java.util.*; public class DateCourante { public static void main(String[] args) { // première manière long t = System.currentTimeMillis() / 1000; System.out.println("maintenant: " + t + " secondes depuis le 1/01/1970"); // deuxième manière Date d = new Date(); System.out.println("maintenant: " + d); // troisième manière String[] jour = { null, "Dimanche", "Lundi", "Mardi", "Mercredi", "Jeudi", "Vendredi", "Samedi" }; String[] mois = { "janvier", "février", "mars", "avril", "mai", "juin", "juillet", "août", "septembre", "octobre", "novembre", "décembre" }; Calendar c = Calendar.getInstance(); System.out.println("maintenant: " + c.get(Calendar.HOUR) + " heures " + c.get(Calendar.MINUTE) + ", le " + jour[c.get(Calendar.DAY_OF_WEEK)] + " " + c.get(Calendar.DAY_OF_MONTH) + " " + mois[c.get(Calendar.MONTH)] + " " + c.get(Calendar.YEAR)); // quatrième manière (deux exemples) DateFormat f = new SimpleDateFormat(); System.out.println("maintenant: " + f.format(d)); f = new SimpleDateFormat("dd MMMMM yyyy HH:mm"); System.out.println("maintenant: " + f.format(d)); } } A l’exécution on obtient : maintenant: 1100428319 secondes depuis le 1/01/1970 maintenant: Sun Nov 14 11:31:59 CET 2004 maintenant: 11 heures 31, le Dimanche 14 novembre 2004 maintenant: 14/11/04 11:31 maintenant: 14 novembre 2004 11:31 La troisième manière est la plus souple, mais la plus laborieuse. Une qualité de la quatrième manière est qu’elle intègre les particularités locales. Ainsi, par défaut, on obtient 14/11/04 11:31, l’affichage court « à la française ». 1.7. Lectures simples au clavier : la classe Scanner import java.util.Scanner; public class TestScanner { public static void main(String[] args) { Scanner entree = new Scanner(System.in); int nombre = 0; float somme = 0; System.out.print("Donner les nombres (terminer par -1): "); float x = entree.nextFloat(); while (x >= 0) { somme += x; nombre++; x = entree.nextFloat(); } System.out.println("moyenne: " + somme / nombre); } } Simplement pour connaître l'équivalent de la fonction python input() . Peu utile en Java, car les entrées sont passées soit par args , soit grâce à une interface graphique. uploads/s1/ exo01-corrige.pdf
Documents similaires
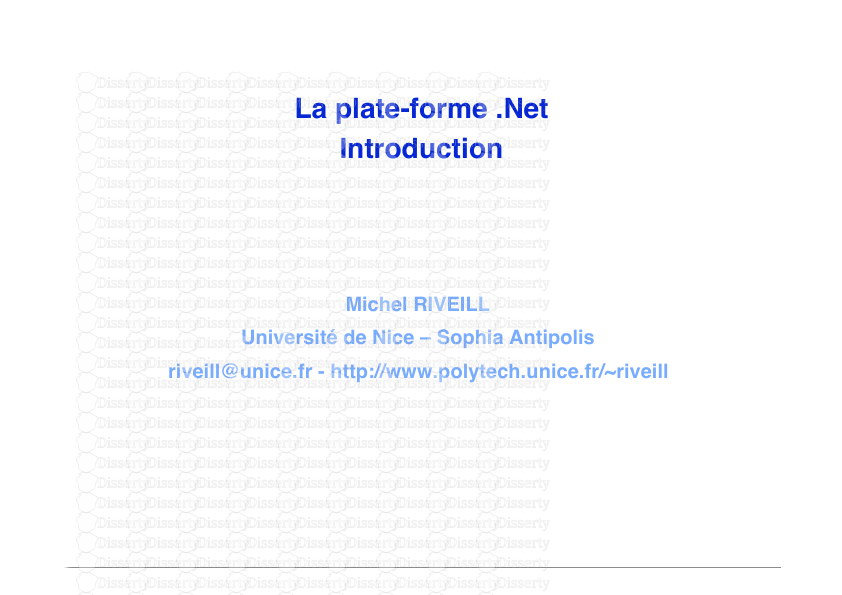
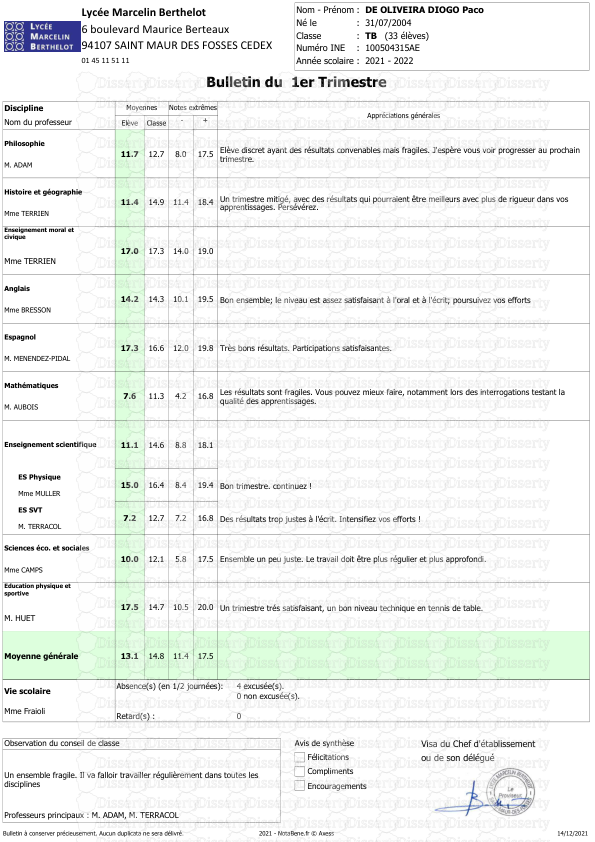
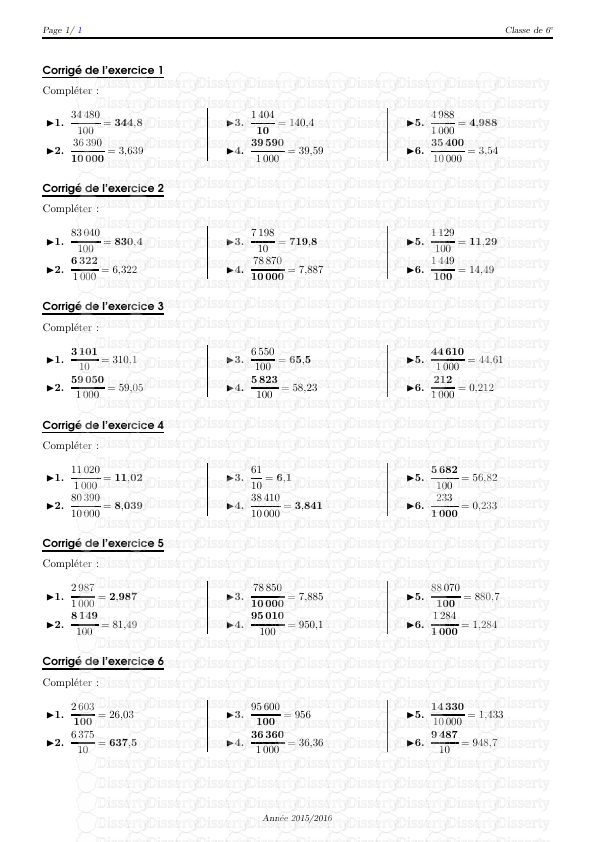
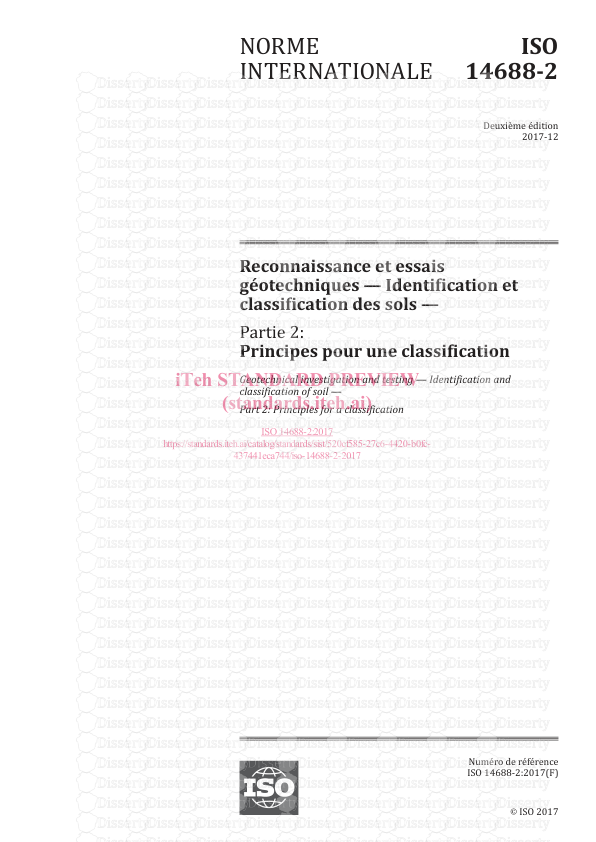
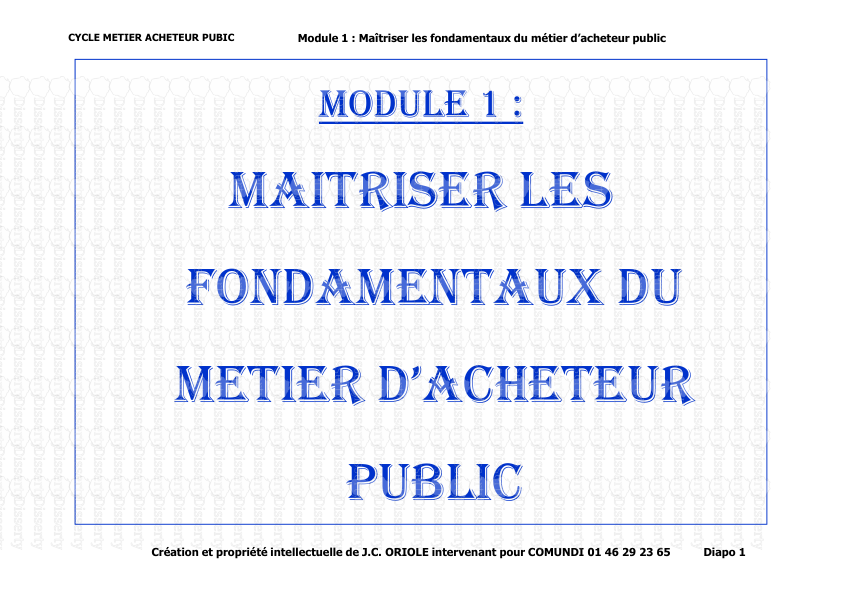
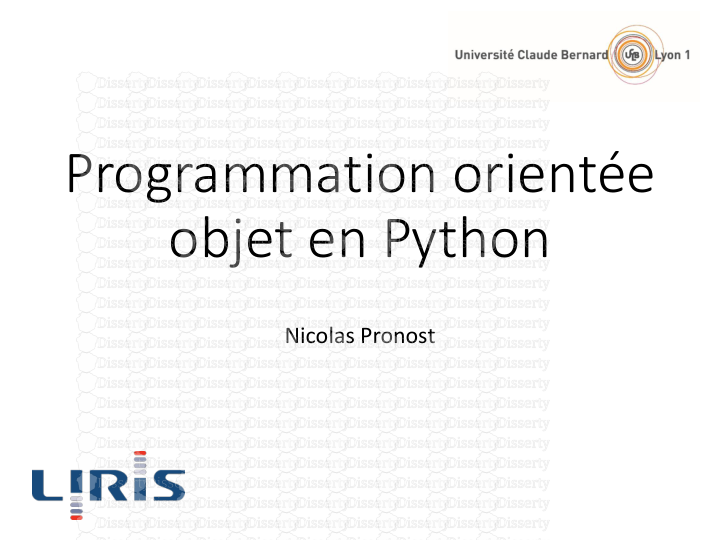
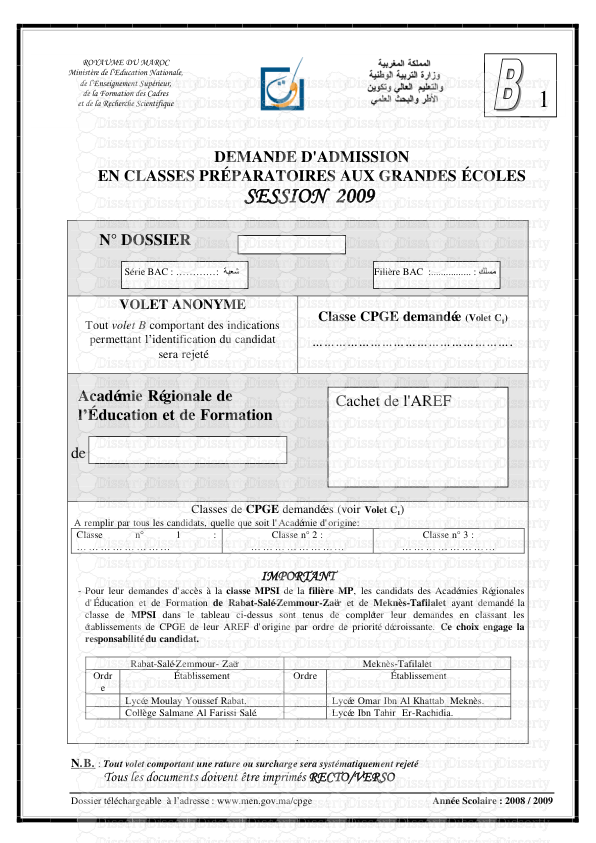
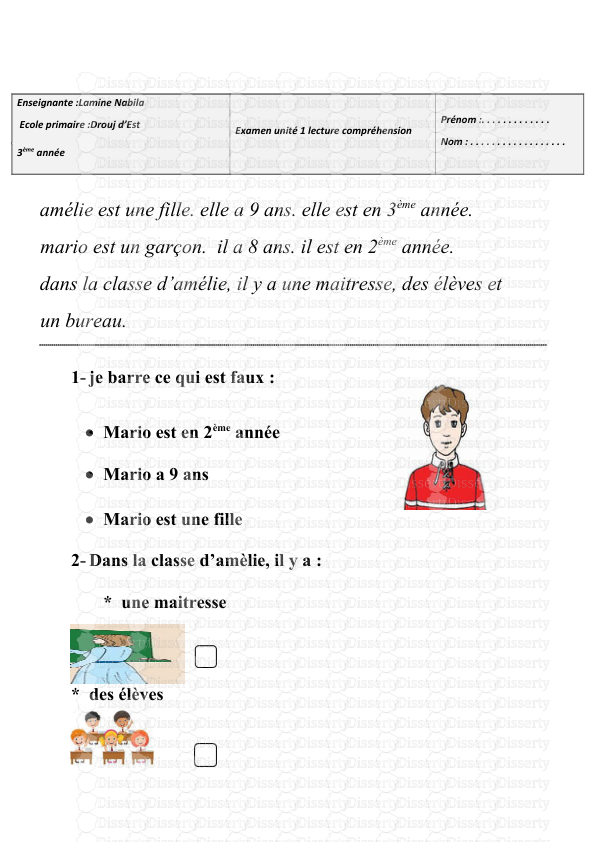
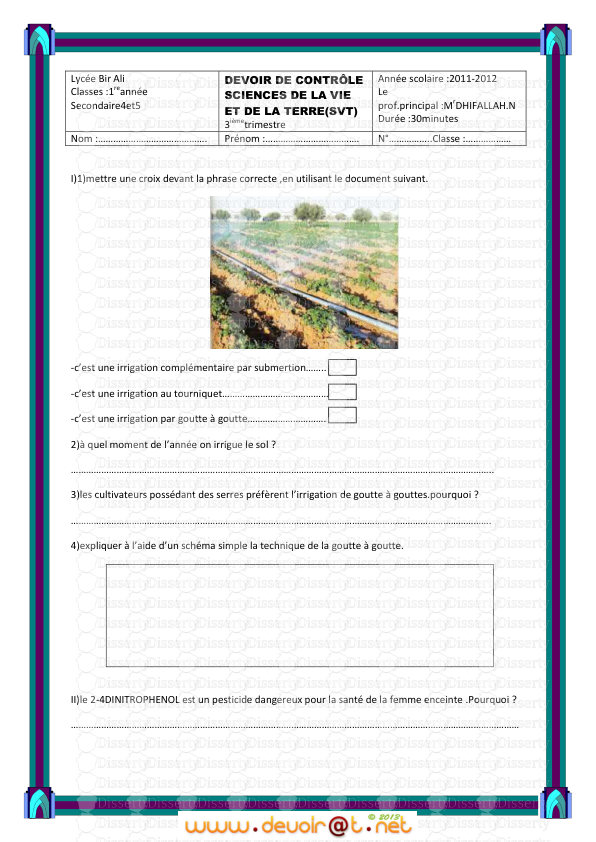
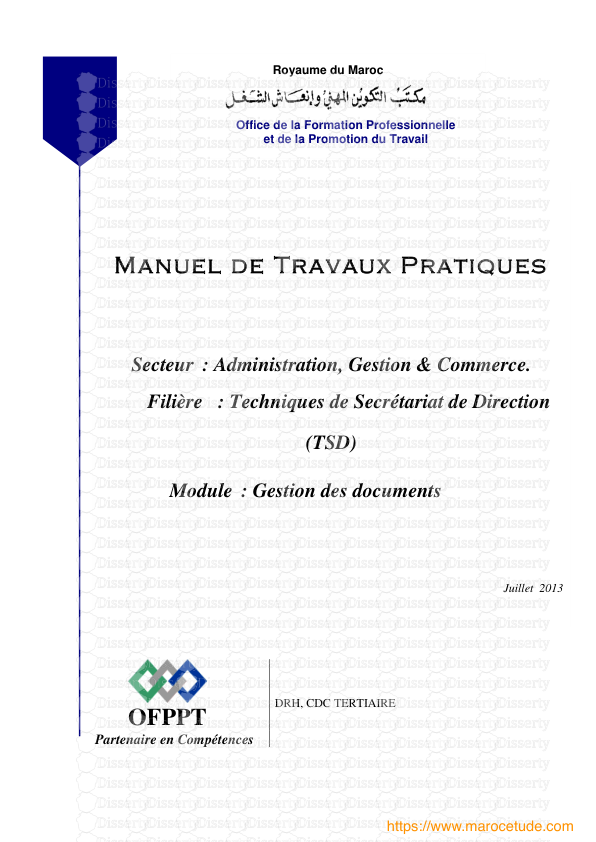
-
31
-
0
-
0
Licence et utilisation
Gratuit pour un usage personnel Attribution requise- Détails
- Publié le Nov 07, 2022
- Catégorie Administration
- Langue French
- Taille du fichier 0.1236MB