1. Introduction 2. let + const 3. arrow functions 4. default + rest + spread 5.
1. Introduction 2. let + const 3. arrow functions 4. default + rest + spread 5. destructuring 6. strings 7. iterators 8. generators 9. classes and inheritance 10. modules 11. promises 12. set, map, weak Table of Contents es6-guide 2 ECMAScript 6 (ES6) guide CHECK SUMMARY TO SEE TABLE OF CONTENT I want to share with you some thoughts, snippets of code and tell you a little about the upcoming ES6. It’s my own road to know it before it will be a standard. You might have noticed about ES6 a lot lately. This is because the standard is targeting ratification in June 2015. See draft - ECMAScript 2015 ECMAScript 2015 is a significant update to the language. Previous (ES5) was standardized in 2009. Frameworks like AngularJS, Aurelia, ReactJS, Ionic start using it today. ES6 includes a lot of new features: arrows classes enhanced object literals template strings destructuring default + rest + spread let + const iterators + for..of generators unicode modules module loaders map + set + weakmap + weakset proxies symbols subclassable built-ins promises math + number + string + object APIs binary and octal literals reflect api ES6-guide es6-guide 3 Introduction tail calls I will try to describe each of these in the next stories, so stay updated. Thanks to the use of transpilers (Babel, Traceur and others) we can actually use it right now until browsers fully catch up. Browser support matrix ES6 Repl in Chrome Devl Tools - Scratch JS Future is bright. People from the community denounced these words. I have only one to add: we can handle it easily! es6-guide 4 Introduction First topic about ECMAScript 2015 is let + const. If you are familiar with JavaScript, you have probably known the term: scope. If you are not that lucky, don't worry about it. I'll explain that in a few words below. Why I mentioned something about JavaScript scope? This is because let and const have a very strong connection with that word. Firstly, imagine and old way (and still valid) to declare a new variable in your JS code using ES5: // ES5 var a = 1; if (1 === a) { var b = 2; } for (var c = 0; c < 3; c++) { // … } function letsDeclareAnotherOne() { var d = 4; } console.log(a); // 1 console.log(b); // 2 console.log(c); // 3 console.log(d); // ReferenceError: d is not defined // window console.log(window.a); // 1 console.log(window.b); // 2 console.log(window.c); // 3 console.log(window.d); // undefined 1. We can see that variable a is declared as global. Nothing surprising. 2. Variable b is inside an if block, but in JavaScript it doesn't create a new scope. If you are familiar with other languages, you can be disappointed, but this is JavaScript and it works as you see. 3. The next statement is a for loop. C variable is declared in this for loop, but also in the global scope. 4. Until variable d is declared in his own scope. It's inside a function and only function creates new scopes. Variables in JavaScript are hoisted to the top! Hoisting is JavaScript's default behavior of moving all declarations to the top of the current scope (to the top of the current script or the current function). In JavaScript, a variable can be declared after it has been used. In other words - a variable can be used before it has been declared! One more rule, more aware: JavaScript only hoists declarations, not initialization. // scope and variable hoisting var n = 1; (function () { console.log(n); var n = 2; let + const es6-guide 5 let + const console.log(n); })(); console.log(n); Let's look for the new keywords in JavaScript ECMAScript 2015: let and const. We can imagine that let is a new var statement. What is the difference? let is block scoped. Let's see an example: // ES6 — let let a = 1; if (1 === a) { let b = 2; } for (let c = 0; c < 3; c++) { // … } function letsDeclareAnotherOne() { let d = 4; } console.log(a); // 1 console.log(b); // ReferenceError: b is not defined console.log(c); // ReferenceError: c is not defined console.log(d); // ReferenceError: d is not defined // window console.log(window.a); // 1 console.log(window.b); // undefined console.log(window.c); // undefined console.log(window.d); // undefined As we can see, this time only variable a is declared as a global. let gives us a way to declare block scoped variables, which is undefined outside it. I use Chrome (stable version) with #enable-javascript-harmony flag enabled. Visit chrome://flags/#enable- javascript-harmony, enable this flag, restart Chrome and you will get many new features. You can also use BabelJS repl or Traceur repl and compare results. const is single-assignment and like a let, block-scoped declaration. // ES6 const { const PI = 3.141593; PI = 3.14; // throws “PI” is read-only } console.log(PI); // throws ReferenceError: PI is not defined const cannot be reinitialized. It will throw an Error when we try to assign another value. let const es6-guide 6 let + const Let's look for the equivalent in ES5: // ES5 const var PI = (function () { var PI = 3.141593; return function () { return PI; }; })(); es6-guide 7 let + const A new syntactic sugar which ES6 brings us soon, called arrow functions (also known as a fat arrow function). It's a shorter syntax compared to function expressions and lexically binds this value. REMEMBER - Arrow functions are always anonymous. How does it look? It's a signature: ([param] [, param]) => { statements } param => expression (param1, param2) => { block } ..and it could be translated to: () => { … } // no argument x => { … } // one argument (x, y) => { … } // several arguments x => { return x * x } // block x => x * x // expression, same as above Lambda expressions in JavaScript! Cool! Instead of writing: [3, 4, 5].map(function (n) { return n * n; }); ..you can write something like this: [3, 4, 5].map(n => n * n); Awesome. Isn't it? There is more! The value of this inside of the function is determined by where the arrow function is defined not where it is used. No more bind, call and apply! No more: var self = this; arrow functions Syntactic sugar Fixed "this" = lexical "this" es6-guide 8 arrow functions It solves a major pain point (from my point of view) and has the added bonus of improving performance through JavaScript engine optimizations. // ES5 function FancyObject() { var self = this; self.name = 'FancyObject'; setTimeout(function () { self.name = 'Hello World!'; }, 1000); } // ES6 function FancyObject() { this.name = 'FancyObject'; setTimeout(() => { this.name = 'Hello World!'; // properly refers to FancyObject }, 1000); } typeof returns function instanceof returns Function It's cannot be used as a constructor and will throw an error when used with new. Fixed this means that you cannot change the value of this inside of the function. It remains the same value throughout the entire lifecycle of the function. Regular functions can be named. Functions declarations are hoisted (can be used before they are declared). The same function Limitations es6-guide 9 arrow functions ECMAScript 2015 functions made a significant progress, taking into account years of complaints. The result is a number of improvements that make programming in JavaScript less error-prone and more powerful. Let's see three new features which give us extended parameter handling. It's a simple, little addition that makes it much easier to handle function parameters. Functions in JavaScript allow any number of parameters to be passed regardless of the number of declared parameters in the function definition. You probably know commonly seen pattern in current JavaScript code: function inc(number, increment) { // set default to 1 if increment not passed // (or passed as undefined) increment = increment || 1; return number + increment; } console.log(inc(2, 2)); // 4 console.log(inc(2)); // 3 The logical OR operator (||) always returns the second operand when the first is falsy. ES6 gives us a way to set default function parameters. Any parameters with a default value are considered to be optional. ES6 version of inc function looks like this: function inc(number, increment = 1) { return number + increment; } console.log(inc(2, 2)); // 4 console.log(inc(2)); // 3 You can also set default values to parameters that appear before arguments without default values: function sum(a, b = 2, c) { return a + b + c; } console.log(sum(1, 5, 10)); // 16 -> b === 5 console.log(sum(1, undefined, 10)); // 13 -> b as default You can even execute a function to set default parameter. It's not restricted to primitive values. function getDefaultIncrement() { return 1; } function inc(number, increment = getDefaultIncrement()) { return number + increment; } default + rest + spread default es6-guide 10 default + rest + spread console.log(inc(2, 2)); // 4 console.log(inc(2)); // 3 Let's uploads/S4/ es6-guide.pdf
Documents similaires
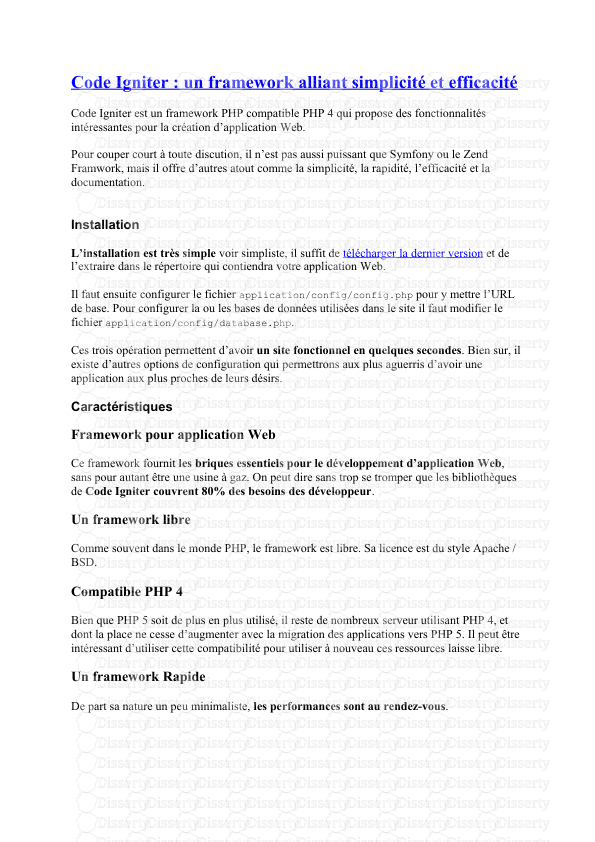
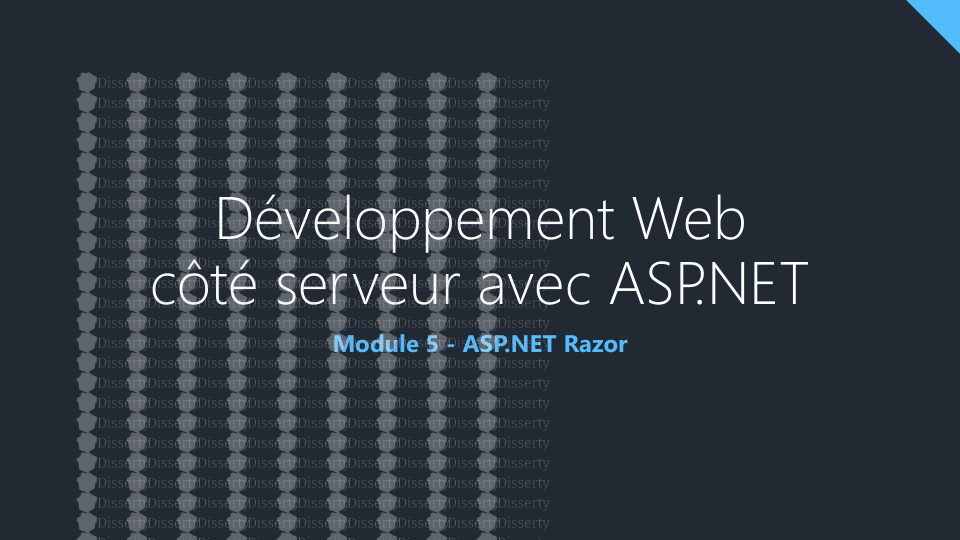
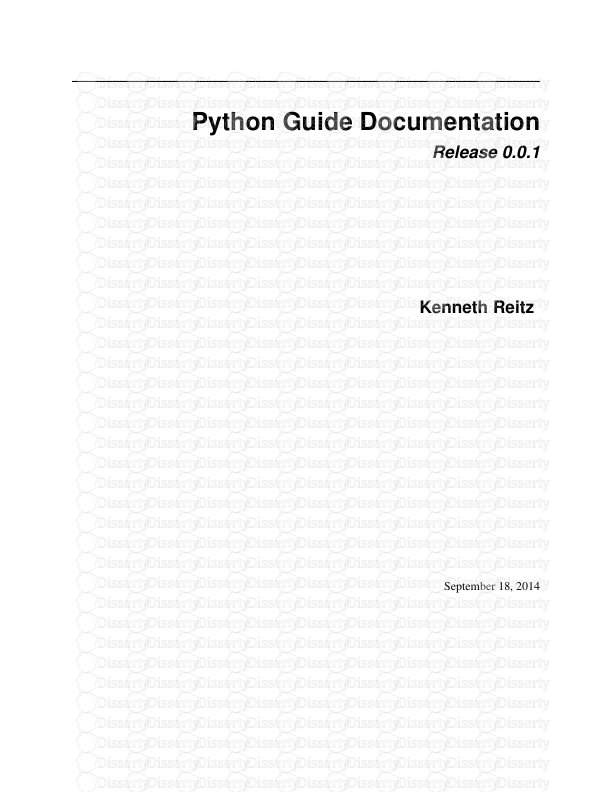
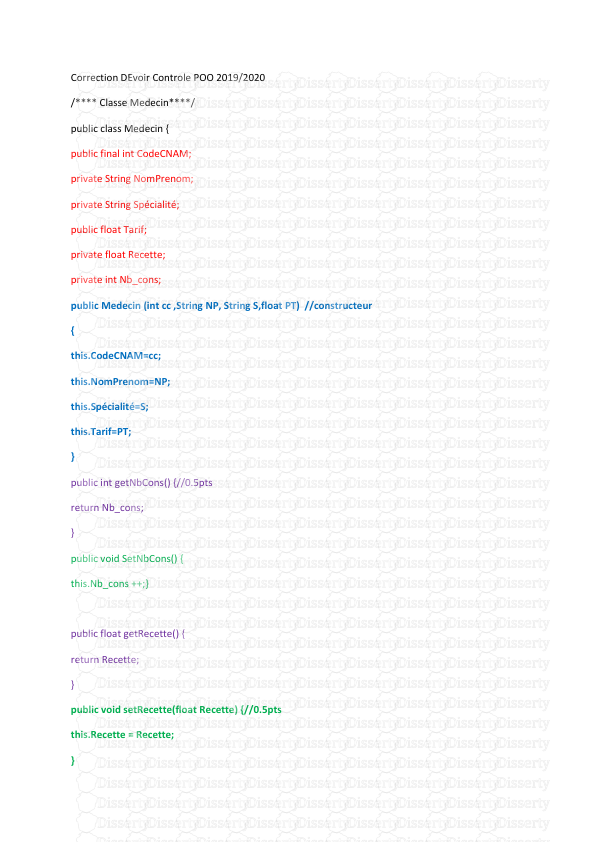
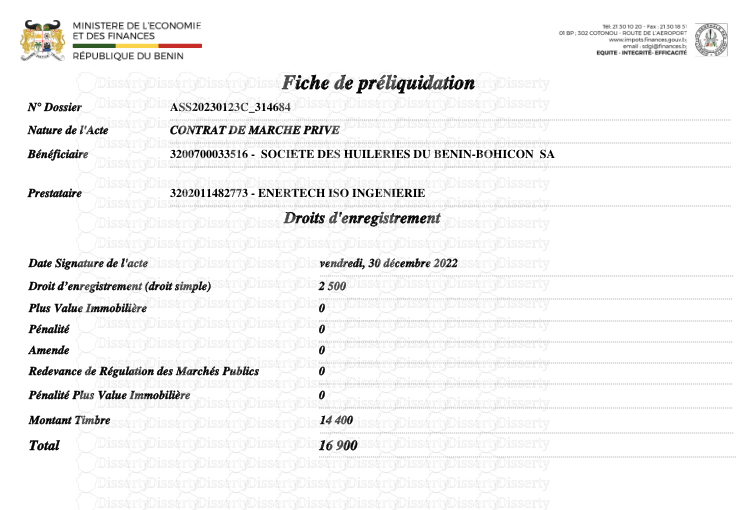
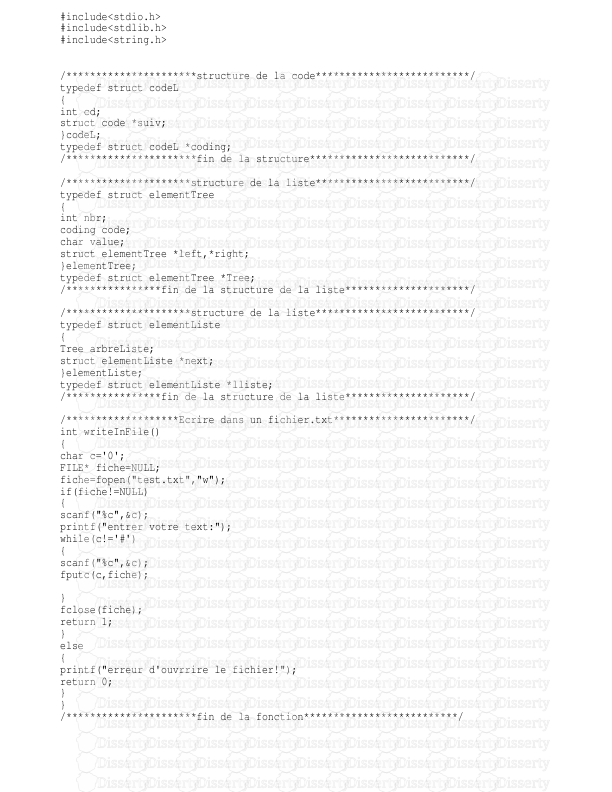
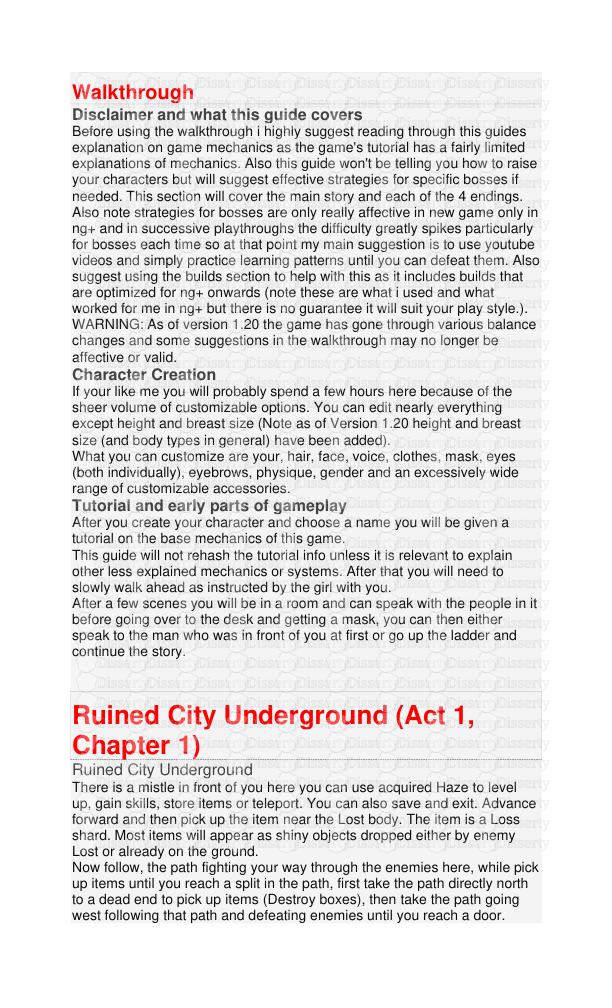
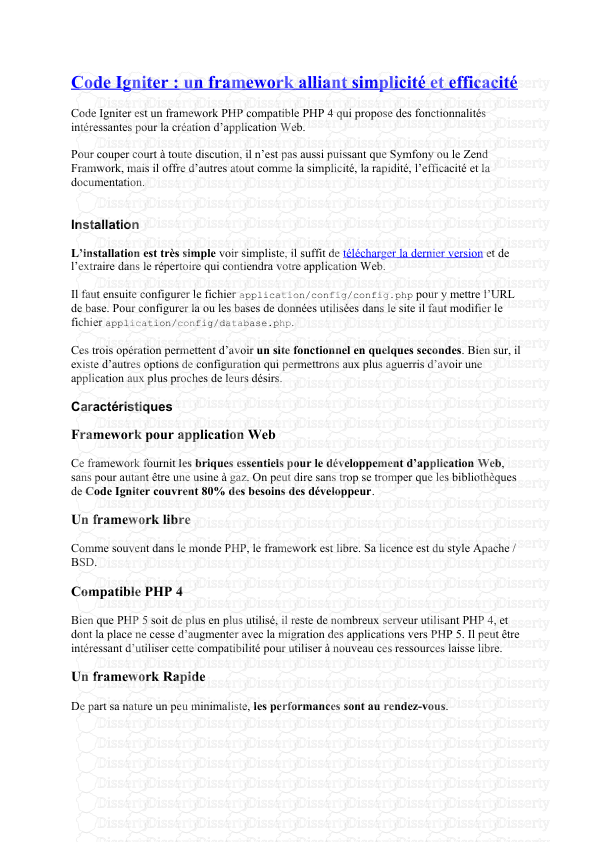
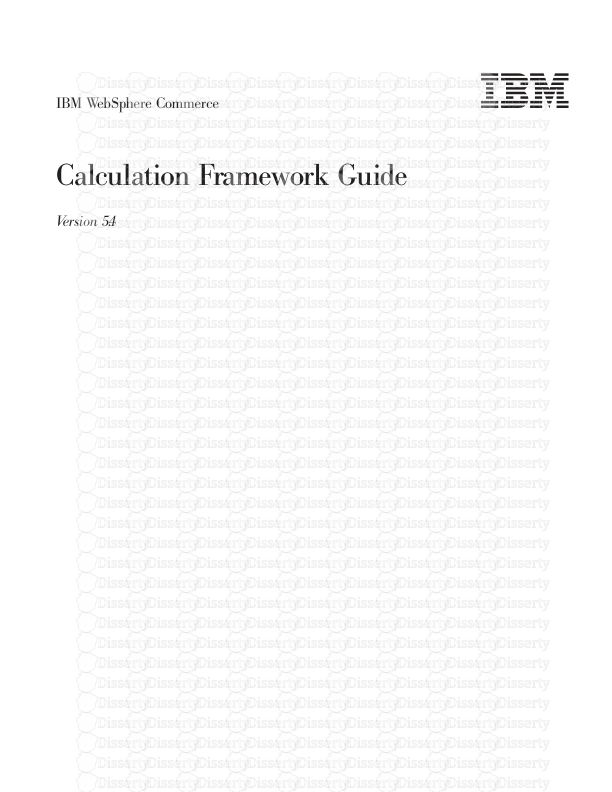
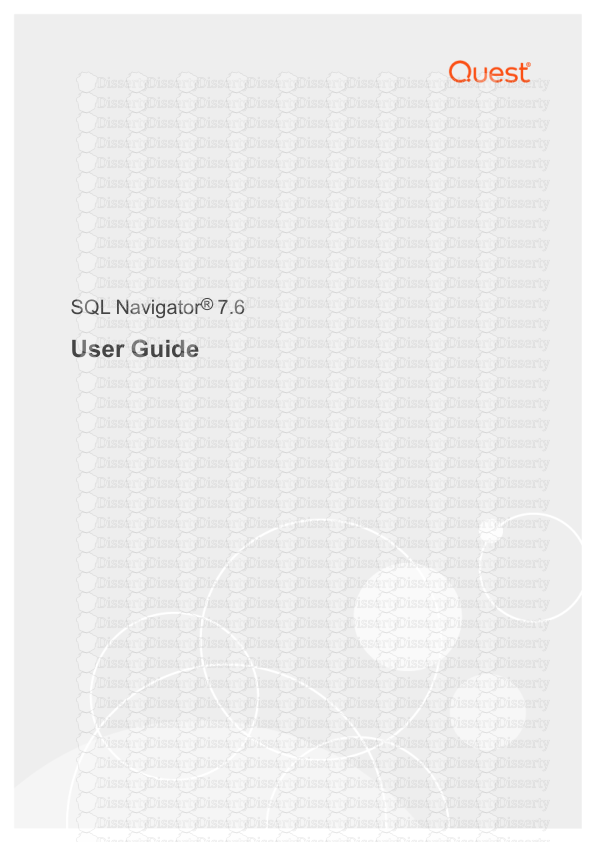
-
43
-
0
-
0
Licence et utilisation
Gratuit pour un usage personnel Attribution requise- Détails
- Publié le Nov 28, 2022
- Catégorie Law / Droit
- Langue French
- Taille du fichier 0.4275MB