© Bjarne Stroustrup, 2008-2009 D-R-A-F-T Instructor’s notes D-R-A-F-T 1 4/7/200
© Bjarne Stroustrup, 2008-2009 D-R-A-F-T Instructor’s notes D-R-A-F-T 1 4/7/2009 Instructor’s Guide for Programming Principles and Practice using C++ Bjarne Stroustrup Texas A&M University http://www.research.att.com/~bs bs@cs.tamu.edu Abstract This is a grab-bag of observations and information that might be helpful if you run a course based on “Programming: Principles and Practice using C++”. Assumptions I assume that you are a professor, lecturer, instructor, teaching assistant, or whatever teaching or about to teach a course based on “Programming: Principles and Practice using C++.” I assume that you have read (at least) the book’s preface and Chapter 0 “Notes to the reader.” If you have not, please do so before proceeding. I assume that your students have never programmed before, have a weak programming background, or have programmed in a language different from C++. I mostly address issues related to the first two groups. After the general information, I have comments relating to individual chapters. I write those comments to be read while planning a lecture based on that chapter. My slides are available (www.stroustrup.com/Programming); if you use them, I hope you’ll suggest improvements. Feedback and descriptions of teaching experiences are most welcome. Note: These are notes, just notes. Don’t expect book quality copyediting. Don’t expect completeness. Do expect updates based on further experience and more time spent on these notes. I plan to keep updating this guide (available on the support site). © Bjarne Stroustrup, 2008-2009 D-R-A-F-T Instructor’s notes D-R-A-F-T 2 4/7/2009 General The greatest obstacle to learning to write good programs is a firm belief that • it is among the most difficult of technical skills • it is a skill that requires some rare talent • it is done by socially inept young men in total isolation, mostly by night • it is mostly about building violent video games • it is a skill that requires mastery of advanced mathematics • it is an activity completely different from everyday ways of thinking • it is something that doesn’t help people As a professor, teacher, instructor, teaching assistant, etc. it is your most important task to minimize the impact of these myths. One thing we have done, which appears to be successful, is to take a couple of minutes at the start of some lectures (maybe every third or fourth) to briefly tell about some interesting application of programming (Chapter 1 gives an idea of what we find interesting). The point is to make the otherwise dry programming material appear relevant by pointing to where it applies. We consider it important that these comments are brief and not too preachy. Unless you fell something is exciting, don’t try to tell students that it is. Students are experts at detecting forced enthusiasm. We typically base these brief presentations/explanations of our personal experience: something we saw on a trip, found in the news, or read in a magazine. A photo or two (or an actual gadget, e.g. an iPod, a cell phone, a watch) can be important as a prop. Please note that not every student find the same type of news/use interesting. A new development in video games may be exciting to some students, but can also reinforce a negative image of programming to many students who are not quite sure whether programming/CS/IT is for them. We find biological and biomedical applications interest that second group of students disproportionately. It is essential to provide a great variety of applications or the students nod off and retain a narrow and generally unimaginative view of software. Wherever possible, try to draw a direct connection from the example to the code: “Google uses techniques just like the STL” is a good example for the STL chapters, the iPod interface for the GUI chapter, and an airplane for the access to hardware and correctness sections, etc. It is essential that students get the idea that writing and running small programs are part of “reading a chapter”. We find that for many freshmen, some material in the first few chapters seems too easy to take seriously and some parts too esoteric to bother with. The students often come from a background where they have either done a fair bit of programming and think they know everything (at least everything that anyone could possibly tell them during the first month). That view may even make them skip early lectures. Others have done no programming and think that they can learn everything from reading a book. In either case, they have the impression from high school that they know what is important and what is not. Some of the best students also underestimate the time and effort they need to spend – often they were doing really well in high school without much effort. Reality tends to be different. The sooner this reality hits home the better. © Bjarne Stroustrup, 2008-2009 D-R-A-F-T Instructor’s notes D-R-A-F-T 3 4/7/2009 Of all the things that correlate with success in this course, “spending the time” is the most important; not previous programming experience, previous grades, or brainpower (as far as we can tell). The drills are there to get people a minimal acquaintance with reality, but attending the lectures is essential, and doing some exercises really matter. We use “Labs” and teaching assistants to ensure the latter. Studying in small groups – preferably heterogonous (in terms of programming background) – groups is another indicator of success. So please try very hard to for each chapter get every student to: 1. Do the “Drill.” It is good to have an instructor, TA, or experienced student accessible to help with “small practical problems” that can spring up unpredictably. 2. Read the “Review” and answer at least a few of the questions. This may require re-reading some part of the text. Good students will do this naturally. Some of the students who need it most will need “prodding.” 3. Do a couple or more exercises. The exercises are roughly ordered according to difficulty (easiest first) and later exercises sometimes build on earlier ones. Beware of geeky know-it-alls who try to convince other students that they are smart by referring back to their high-school wisdom or what they found in web-forums. The most damaging “bright idea” is to convince other students that arrays and pointers are much better than vectors and strings, thus diverting genuine naïve students into the problems of range errors, fixed size allocations, strcpy() use, etc. long before they are ready for that. It have been suggested that we write for “elite students at elite institutions”. That is of course very complimentary to TAMU’s freshmen, but not realistic. We are not among the most selective colleges and the students were not all as highly motivated for this course as we might have liked. In fact, the first several hundred students were mostly EE and Computer Engineering students who did not see software as central to their studies (and the course is compulsory). Only later, after seeing very positive results compared with the CS majors taking a traditional first CS course, did we include CS freshmen. If you are teaching a large class, not everyone will pass/succeed. In that case you have a choice which in its crudest for is: slow down to help the weaker students or keep up the pace and lose them. The urge and pressure is typically to slow down and help. By all means help –and supply extra help through teaching assistants if you can – but don’t slow down. Doing so would not be fair to the smartest, best prepared, and hardest working students – you’ll lose them to boredom and lack of challenge. If you have to lose/fail someone, let it be someone that will never become a good software developer or computer scientist anyway; not your potential star students. Computer Science and software development are losing students to fields of study perceived to be more challenging. Please don’t add to that problem. We strongly believe that students live up (or down) to the expectations made of them, and this course seems to bear it out. Surveys show the students in this course work 25% more hours and report 25% more positive opinion than the average freshman course (incidentally, that was the highest satisfaction score in the college of engineering). This course is no harder than average freshman biology, physics, or math freshman course, © Bjarne Stroustrup, 2008-2009 D-R-A-F-T Instructor’s notes D-R-A-F-T 4 4/7/2009 given an ordinary US high school background (where the preparation tends to be better in more established academic fields, such as biology, than in CS). It has been suggested that the course has succeeded “because it was taught by Bjarne Stroustrup.” That again is very complimentary, but not realistic. The freshmen are not awed by reputation and many are initially (sadly) far more interested in grades than actually learning serious technical stuff. Anyway, for the last 4 semesters the course has been taught by Pete Petersen, Walter Daugherity, and Ronnie Ward; they are very experienced teachers of undergraduates; I have just done the odd uploads/Litterature/ instructor-x27-s-guide-programming-bjarne-stroustrup.pdf
Documents similaires
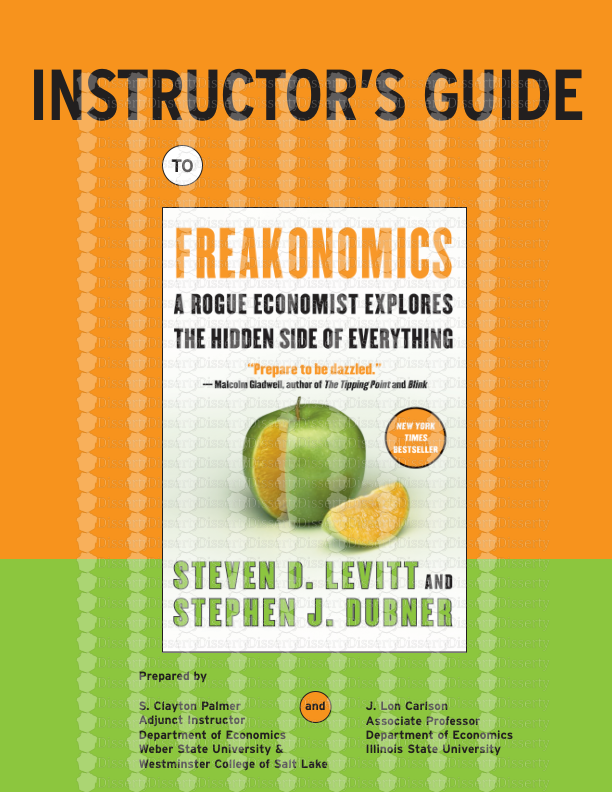
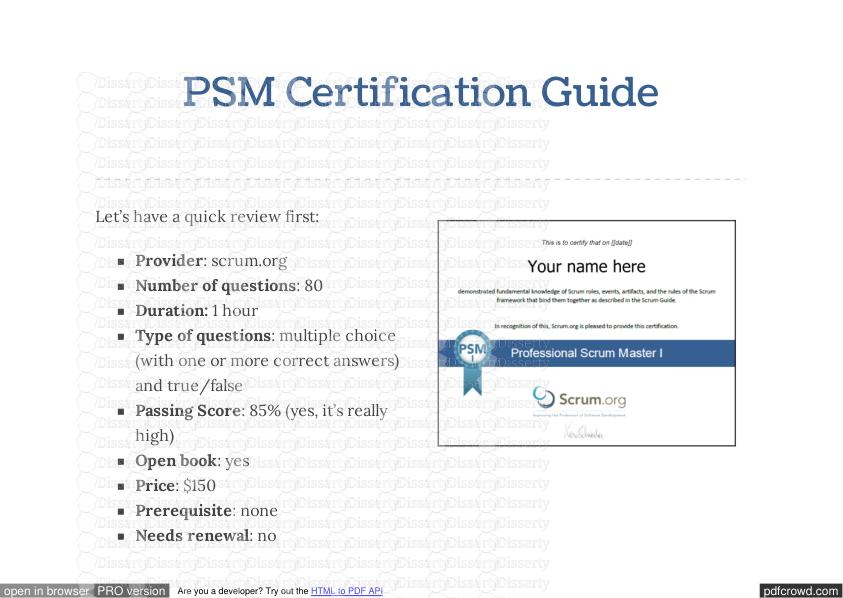
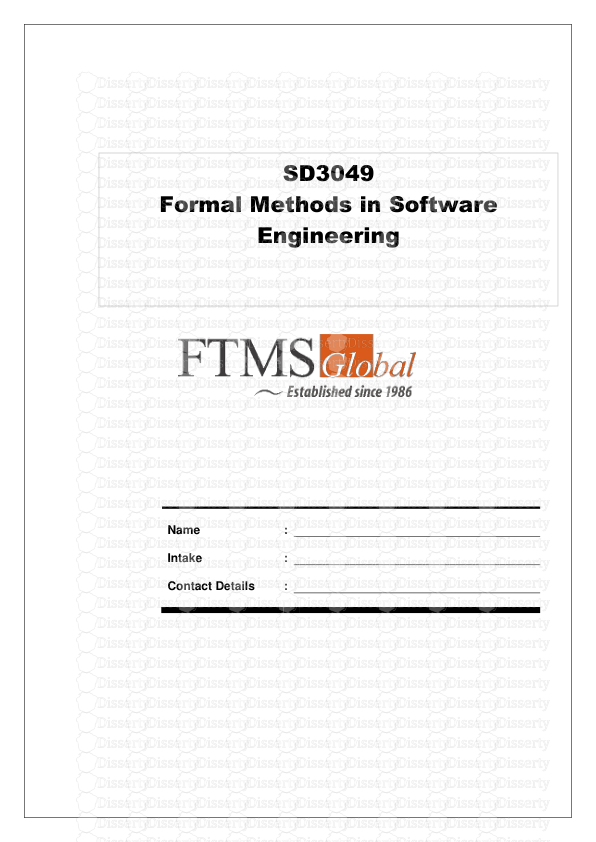
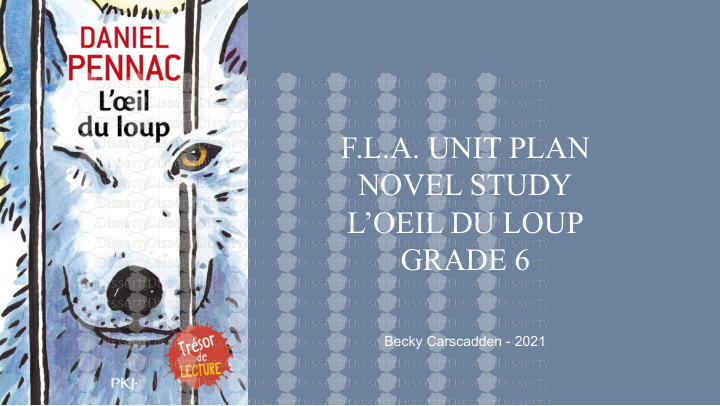
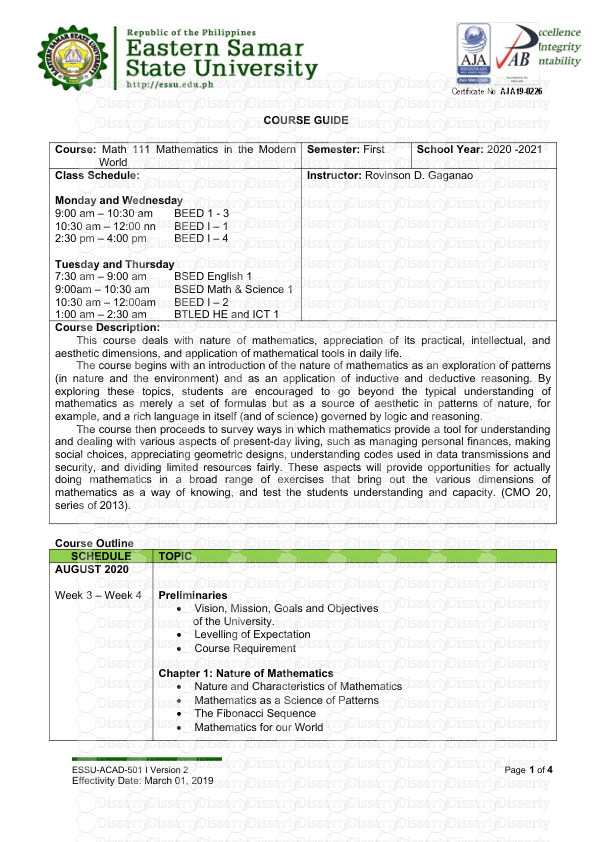
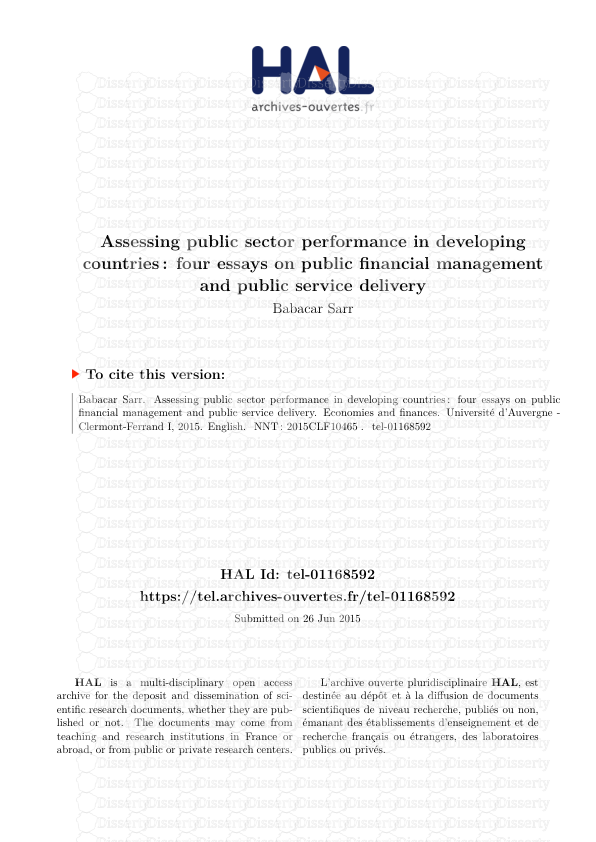
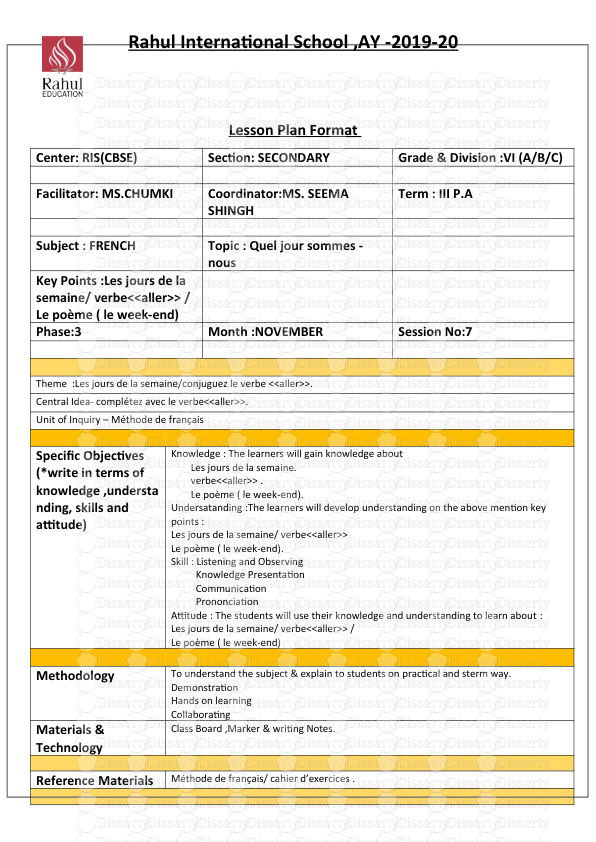
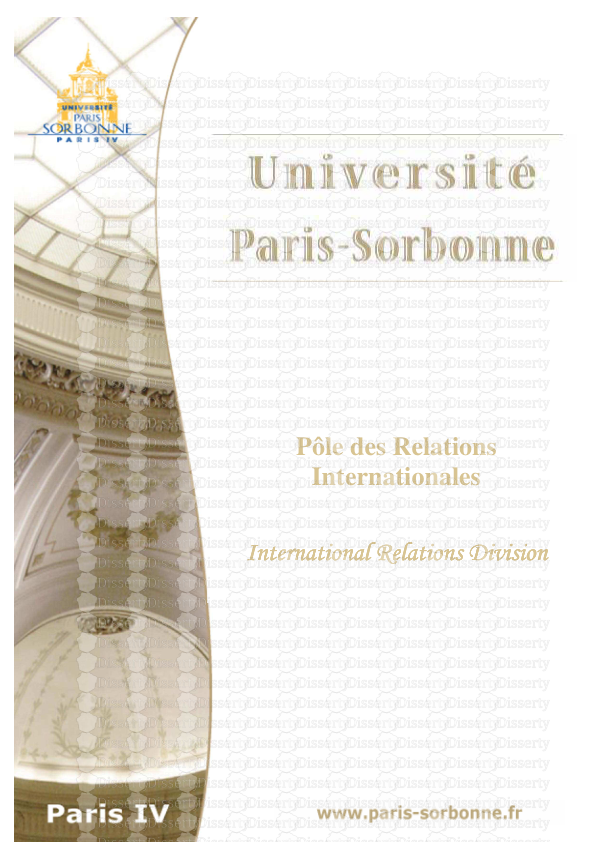
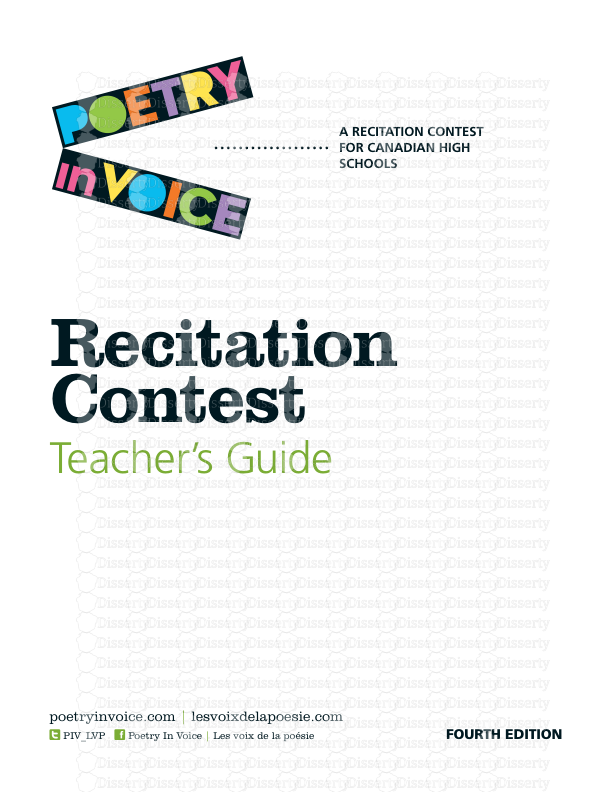
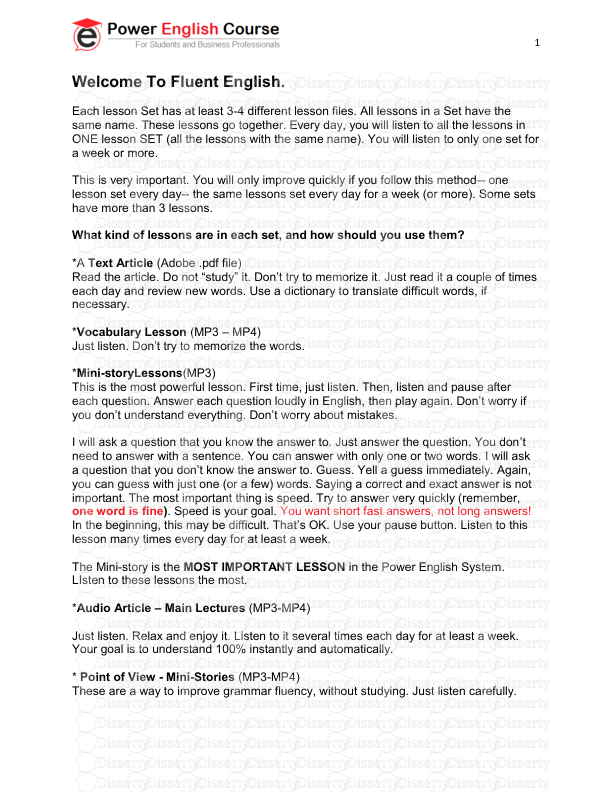
-
51
-
0
-
0
Licence et utilisation
Gratuit pour un usage personnel Attribution requise- Détails
- Publié le Dec 06, 2022
- Catégorie Literature / Litté...
- Langue French
- Taille du fichier 0.2093MB