package ch.vd.sieeo.neo.service.csv; import java.text.DateFormat; import java.t
package ch.vd.sieeo.neo.service.csv; import java.text.DateFormat; import java.text.SimpleDateFormat; import java.util.ArrayList; import java.util.Arrays; import java.util.Calendar; import java.util.Date; import java.util.Iterator; import java.util.List; import java.util.Map; import ch.vd.sieeo.neo.domain.Ecr; import ch.vd.sieeo.neo.domain.EcrObjectif; import ch.vd.sieeo.neo.domain.Eleve; import ch.vd.sieeo.neo.domain.EleveObjectif; import ch.vd.sieeo.neo.domain.Evaluation; /** * @author neo * */ public class CsvEcrDelegate extends CsvDelegate { private Ecr ecr; private List<Eleve> eleves; private Map<Long, Evaluation> evaluations; private List<EcrObjectif> objs; private boolean isNewFile; private Map<EleveObjectif, Evaluation> mapElevObj; public CsvEcrDelegate(Ecr ecr, List<EcrObjectif> objs, Map<EleveObjectif, Evaluation> mapElevObj, Map<Long, Evaluation> evaluations, List<Eleve> eleves, boolean isNewFile) { this.ecr = ecr; this.eleves = eleves; this.evaluations = evaluations; this.objs = objs; this.isNewFile = isNewFile; this.mapElevObj = mapElevObj; } /* * (non-Javadoc) * * @see ch.vd.sieeo.neo.service.csv.CsvDelegate#getHeaders() */ @Override public List<String> getHeaders() { String[] headers = { "ANREF", "LIVREUR", "DATLIVR", "ID_ECR", "ECR_ANNEE", "ECR_VOIE", "ECR_NIVEAU", "ECR_DISCIPLINE", "ECR_TYPE", "ECR_DATEPASSATION", "CLAnn eCourant", "VOIE", "IDCLASSE", "NMCLASSE", "NUMDFJ", "ANNAI", "MOINAI", "JOURNAI", "IDETABL", "NMETABL", "SEXE", "NATCOD", "NATLIB", "LANGCODE", "LANGLIB" }; // Get first part of header List<String> headersList = new ArrayList<String>(); headersList.addAll(Arrays.asList(headers)); // Add objectifs // EDV-NEO-243 : Export should have 6 columns int size = 6; // objs.size(); for (int count = 1; count <= size; count++) { String anObjectifCode = "CD_OBJECTIF" + count; String anObjectifPoint = "Points_Objectif" + count; headersList.add(anObjectifCode); headersList.add(anObjectifPoint); } headersList.add("total_calcul"); headersList.add("statut_epreuve"); return headersList; } @Override public Iterator<List<String>> iterator() { Iterator<List<String>> it = new Iterator<List<String>>() { private Iterator<Eleve> eleveIte = eleves.iterator(); @Override public boolean hasNext() { return eleveIte.hasNext(); } @Override public List<String> next() { Eleve anEleve = eleveIte.next(); return getContentForEleve(anEleve); } @Override public void remove() { // Bad boy throw new UnsupportedOperationException(); } }; return it; } private List<String> getContentForEleve(Eleve eleve) { List<String> content = new ArrayList<String>(); DateFormat formatter = new SimpleDateFormat("yyyy.MM.dd"); Float sumPoints = 0F; // ANREF content.add("" + ecr.getAnneeScolaire()); // LIVREUR content.add("NEO"); content.add("" + formatter.format(ecr.getCreateDate())); // ID_ECR content.add("" + ecr.getId()); // ECR_ANNEE content.add(ecr.getDegre()); // ECR_VOIE content.add("" + ecr.getVoie()); // ECR_NIVEAU content.add(ecr.getNiveau() != null ? ecr.getNiveau().getAbreviation() : ""); // ECR_DISCIPLINE content.add(ecr.getDiscipline().getAbreviation()); // Get Evaluation For ecrId and eleveId Evaluation ecrEval = evaluations.get(eleve.getId()); String epreuveType = "OFFICIEL"; if (ecrEval != null && ecrEval.isSitParticuliere()) { epreuveType = "RATTRAPAGE"; } // ECR_TYPE content.add(epreuveType); Date datePassation = ecr.getDatePassation(); // ECR_DATEPASSATION content.add(formatter.format(datePassation)); // CLAnn eCourant content.add(eleve.getClasse().getDegre()); // VOIE content.add("" + eleve.getClasse().getVoie()); // IDCLASSE content.add("" + eleve.getClasse().getId()); // NMCLASSE content.add(eleve.getClasse().getNom()); Long dfjc = eleve.getDfjc(); String dfjcToString = ""; if (dfjc != null) { dfjcToString = dfjc.toString(); } // NUMDFJ content.add(dfjcToString); // NOMELEVE // content.add(eleve.getNom()); // PRENOMELEVE // content.add(eleve.getPrenom()); Calendar calendar = Calendar.getInstance(); Date dateNaissance = eleve.getDateNaissance(); String year = ""; String month = ""; String day = ""; if (dateNaissance != null) { calendar.setTime(dateNaissance); year += calendar.get(Calendar.YEAR); month = formattingTwoDigit(calendar.get(Calendar.MONTH) + 1); // Month // of // date // is // 0-base day = formattingTwoDigit(calendar.get(Calendar.DAY_OF_MONTH)); } // ANNAI content.add(year); // MOINAI content.add(month); // JOURNAI content.add(day); // IDETABL content.add(eleve.getEtablissement().getId().toString()); // NMETABL content.add(eleve.getEtablissement().getNom()); // SEXE content.add(eleve.getGenre()); // NATCOD content.add(eleve.getCodeNationalite()); // NATLIB content.add(eleve.getLibelleNationalite()); // LANGCOD content.add(eleve.getCodeLangueMaternelle()); // LANGLIB content.add(eleve.getLibelleLangueMaternelle()); int nbObjectifs = 0; for (EcrObjectif objectifEcr : objs) { // Count number of objectifs nbObjectifs++; // CD_OBJECTIF 1..n content.add(objectifEcr.getCode()); EleveObjectif key = new EleveObjectif(eleve.getId(), objectifEcr.getId()); Evaluation evalOjb = mapElevObj.get(key); if (evalOjb != null) { // Points_Objectif 1..n String pts = evalOjb.getNote() != null ? evalOjb.getNote().toString() : null; content.add(pts); if (evalOjb.getNote() != null) { sumPoints += evalOjb.getNote(); } } else { content.add(null); } } // EDV-NEO-243 : Export should have 6 columns if (nbObjectifs < 6) { // Add empty columns for (int i = nbObjectifs + 1; i <= 6; i++) { // Code content.add(null); // Points content.add(null); } } // total_calcul String sumPtsStr = sumPoints != null ? sumPoints.toString() : null; content.add(sumPtsStr); // status_epreuve // TODO to correct later String status = (ecrEval != null && ecrEval.getRemarque() != null && ! ecrEval.getRemarque().equals("null")) ? ecrEval .getRemarque() : null; content.add(status); return content; } private String formattingTwoDigit(int x) { return ((x < 10) ? "0" : "") + x; } /* * (non-Javadoc) * * @see ch.vd.sieeo.neo.service.csv.CsvDelegate#isNewFile() */ @Override public boolean isNewFile() { return this.isNewFile; } } uploads/Management/ new-text-document.pdf
Documents similaires
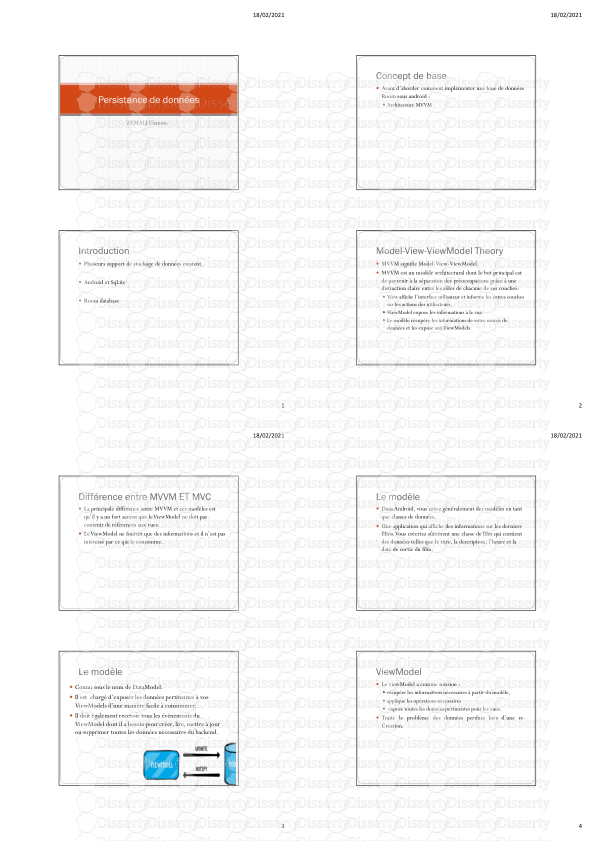
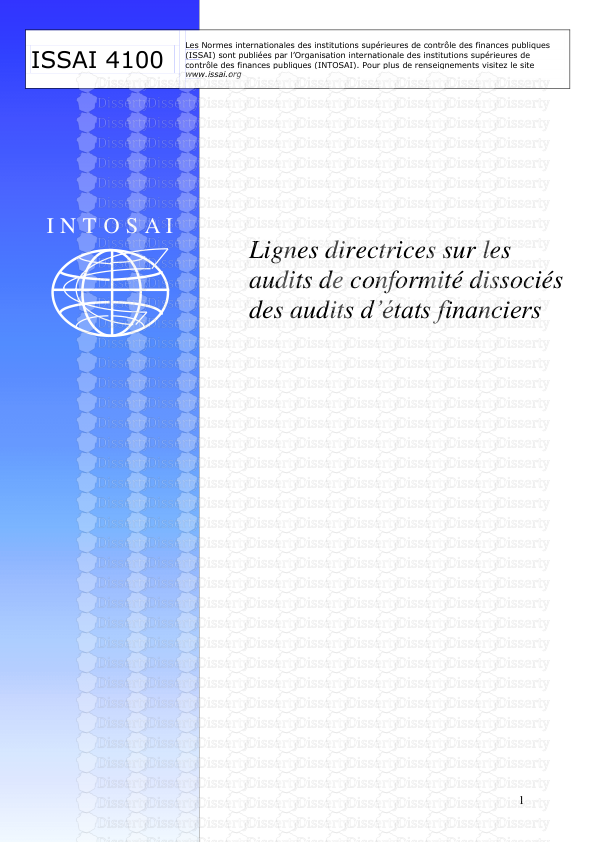
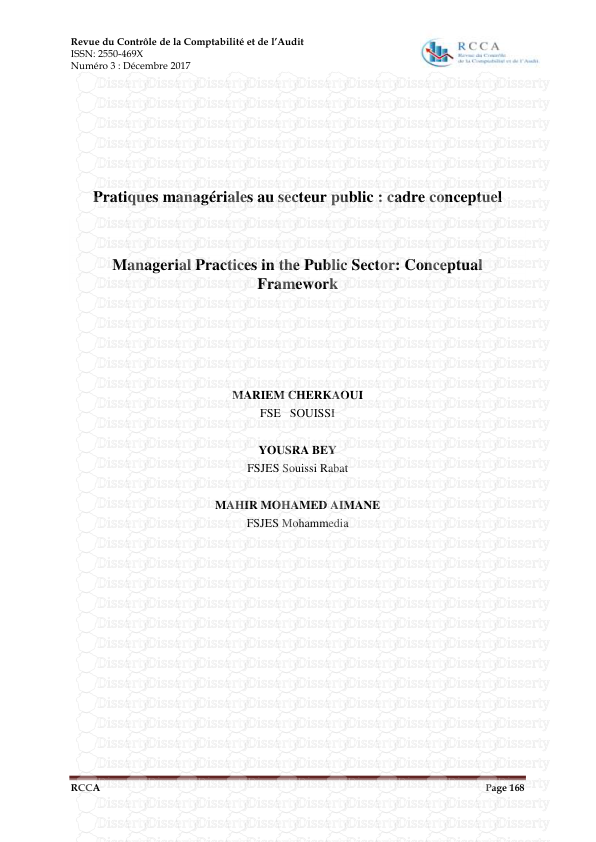
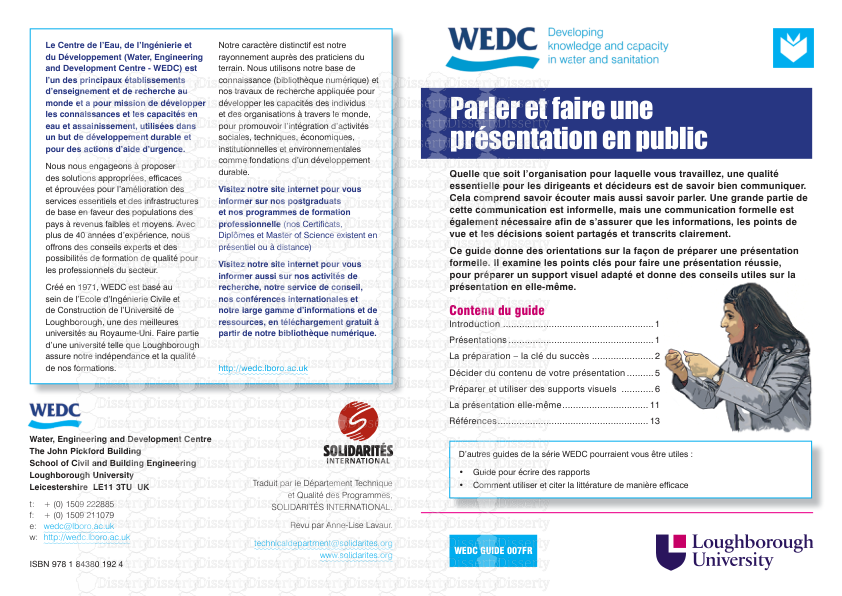
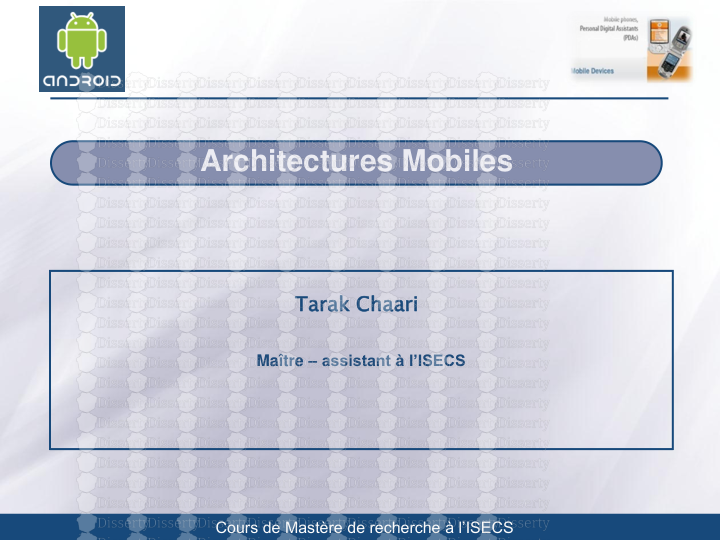
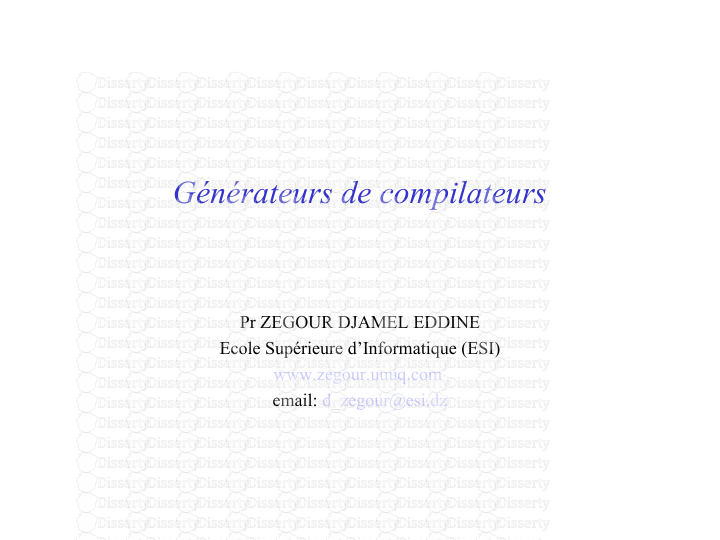
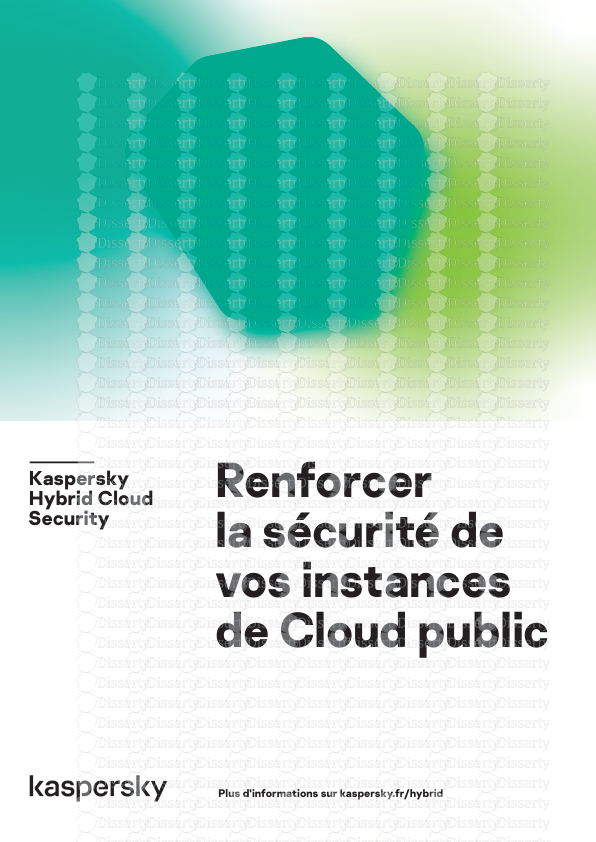
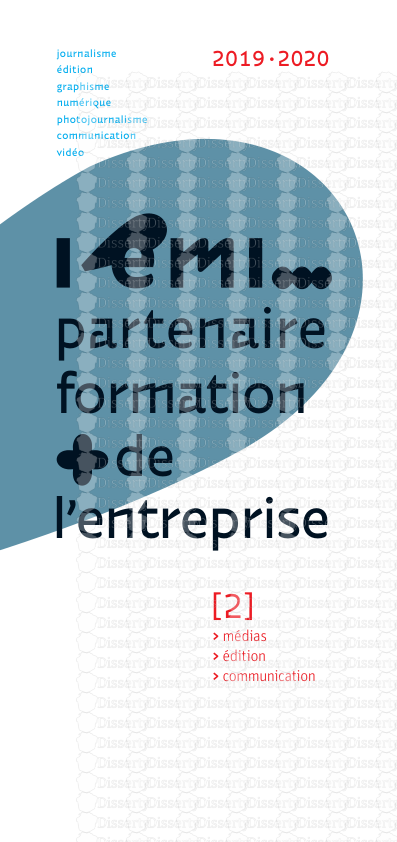
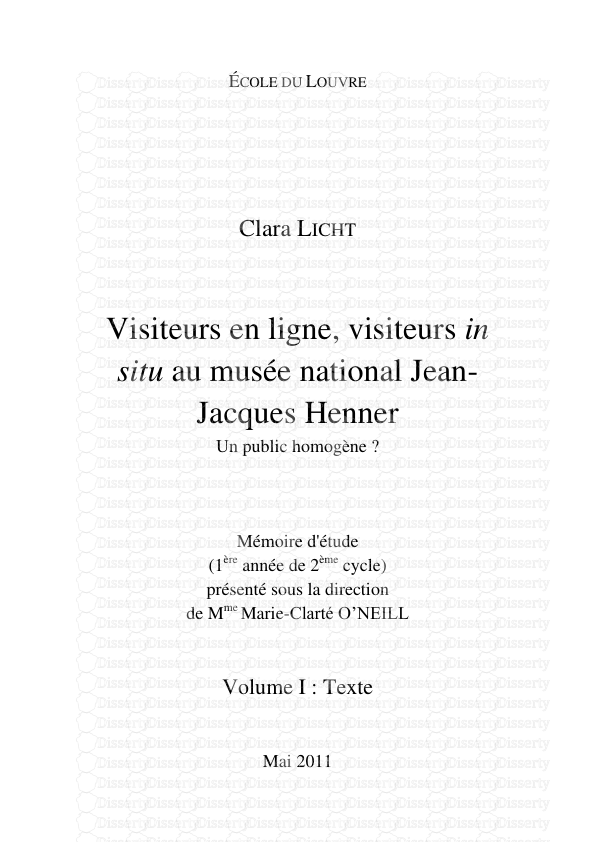
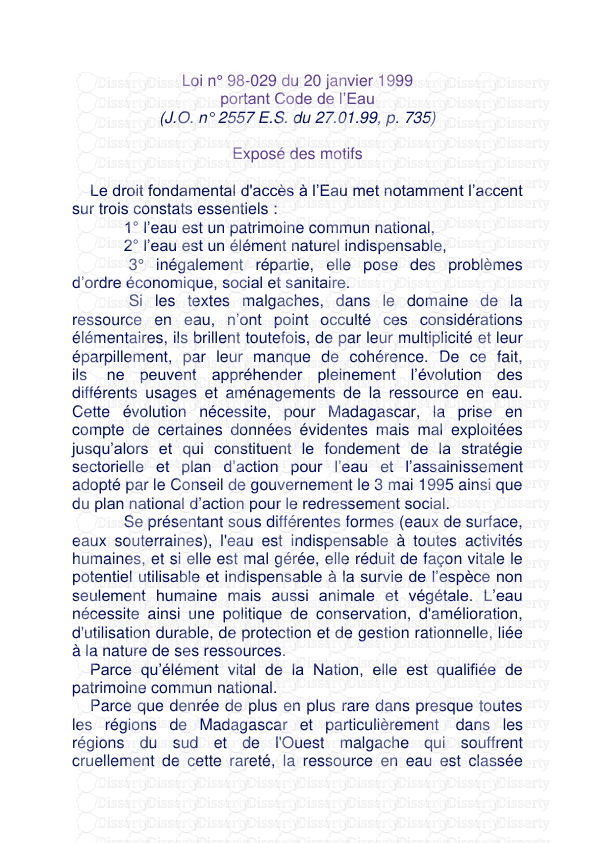
-
22
-
0
-
0
Licence et utilisation
Gratuit pour un usage personnel Attribution requise- Détails
- Publié le Oct 30, 2021
- Catégorie Management
- Langue French
- Taille du fichier 0.0262MB