Advanced Bash−Scripting Guide A complete guide to shell scripting, using Bash M
Advanced Bash−Scripting Guide A complete guide to shell scripting, using Bash Mendel Cooper Brindlesoft thegrendel@theriver.com Revision History Revision 0.1 14 June 2000 Revised by: mc Initial release. Revision 0.2 30 October 2000 Revised by: mc Bugs fixed, plus much additional material and more example scripts. Revision 0.3 12 February 2001 Revised by: mc Another major update. Revision 0.4 08 July 2001 Revised by: mc More bugfixes, much more material, more scripts − a complete revision and expansion of the book. Revision 0.5 03 September 2001 Revised by: mc Major update. Bugfixes, material added, chapters and sections reorganized. This is an major update of version 0.4. The main feature of this release is a major reorganization of the document into parts and chapters (just like a "real" book). Of course, more bugs have been swatted, and additional material and example scripts added. This project has now reached the equivalent of a 360−page book. See the Change.log file for a revision history. This document is both a tutorial and a reference on shell scripting with Bash. It assumes no previous knowledge of scripting or programming, but progresses rapidly toward an intermediate/advanced level of instruction. [1] The exercises and heavily−commented examples invite active reader participation. This project has evolved into a comprehensive book that compares favorably with any of the shell scripting manuals in print. It may serve as a textbook, a manual for self−study, and a reference and source of knowledge on shell scripting techniques. The latest version of this document, as an archived "tarball" including both the SGML source and rendered HTML, may be downloaded from the author's home site. Dedication For Anita, the source of all the magic Table of Contents Chapter 1. Why Shell Programming? ...............................................................................................................1 Chapter 2. Starting Off With a Sha−Bang.......................................................................................................3 2.1. Invoking the script............................................................................................................................5 2.2. Shell wrapper, self−executing script .................................................................................................6 Part 2. Basics .........................................................................................................................................................8 Chapter 3. Exit and Exit Status.........................................................................................................................9 Chapter 4. Special Characters.........................................................................................................................11 Chapter 5. Introduction to Variables and Parameters ..................................................................................24 5.1. Variable Substitution......................................................................................................................24 5.2. Variable Assignment .......................................................................................................................26 5.3. Special Variable Types...................................................................................................................27 Chapter 6. Quoting...........................................................................................................................................31 Chapter 7. Tests................................................................................................................................................37 7.1. Test Constructs...............................................................................................................................37 7.2. File test operators ............................................................................................................................42 7.3. Comparison operators (binary).......................................................................................................44 7.4. Nested if/then Condition Tests.......................................................................................................49 Chapter 8. Operations and Related Topics....................................................................................................51 8.1. Operators .........................................................................................................................................51 8.2. Numerical Constants .......................................................................................................................57 Part 3. Beyond the Basics...................................................................................................................................57 Chapter 9. Variables Revisited........................................................................................................................59 9.1. Internal Variables ............................................................................................................................59 9.2. Parameter Substitution....................................................................................................................73 9.3. Typing variables: declare or typeset..............................................................................................81 9.4. Indirect References to Variables .....................................................................................................83 9.5. $RANDOM: generate random integer ............................................................................................85 9.6. The Double Parentheses Construct.................................................................................................89 Chapter 10. Loops and Branches....................................................................................................................91 10.1. Loops............................................................................................................................................91 10.2. Nested Loops..............................................................................................................................100 10.3. Loop Control ...............................................................................................................................101 10.4. Testing and Branching................................................................................................................103 Chapter 11. Internal Commands and Builtins.............................................................................................109 11.1. Job Control Commands..............................................................................................................124 Chapter 12. External Filters, Programs and Commands...........................................................................128 12.1. Basic Commands........................................................................................................................128 Advanced Bash−Scripting Guide i Table of Contents 12.2. Complex Commands ...................................................................................................................131 12.3. Time / Date Commands..............................................................................................................136 12.4. Text Processing Commands ........................................................................................................138 12.5. File and Archiving Commands...................................................................................................153 12.6. Communications Commands......................................................................................................160 12.7. Terminal Control Commands.....................................................................................................163 12.8. Math Commands .........................................................................................................................164 12.9. Miscellaneous Commands..........................................................................................................167 Chapter 13. System and Administrative Commands..................................................................................173 Chapter 14. Command Substitution.............................................................................................................193 Chapter 15. Arithmetic Expansion ................................................................................................................196 Chapter 16. I/O Redirection ...........................................................................................................................197 16.1. Using exec ...................................................................................................................................199 16.2. Redirecting Code Blocks............................................................................................................199 16.3. Applications................................................................................................................................202 Chapter 17. Here Documents .........................................................................................................................204 Chapter 18. Recess Time................................................................................................................................209 Part 4. Advanced Topics......................................................................................................................209 19.1. A Brief Introduction to Regular Expressions................................................................211 19.2. Globbing........................................................................................................................211 23.1. Complex Functions and Function Complexities ............................................................213 23.2. Local Variables and Recursion......................................................................................215 33.1. Unofficial Shell Scripting Stylesheet .............................................................................218 34.1. Interactive and non−interactive shells and scripts.........................................................220 34.2. Tests and Comparisons..................................................................................................222 34.3. Optimizations .................................................................................................................224 34.4. Assorted Tips.................................................................................................................231 34.5. Portability Issues ............................................................................................................233 37.1. Author's Note.................................................................................................................236 37.2. About the Author...........................................................................................................239 37.3. Tools Used to Produce This Book.................................................................................250 37.3.1. Software and Printware ...............................................................................................251 Bibliography........................................................................................................................................255 Appendix A. Contributed Scripts .........................................................................................................257 Appendix B. A Sed and Awk Micro−Primer .......................................................................................262 B.1. Sed ...................................................................................................................................265 B.2. Awk.................................................................................................................................269 Appendix C. Exit Codes With Special Meanings ................................................................................269 Appendix D. A Detailed Introduction to I/O and I/O Redirection......................................................272 Appendix E. Localization....................................................................................................................272 Appendix F. A Sample .bashrc File.....................................................................................................273 Appendix G. Converting DOS Batch Files to Shell Scripts................................................................273 Advanced Bash−Scripting Guide ii Table of Contents Appendix H. Copyright ........................................................................................................................274 Chapter 19. Regular Expressions..................................................................................................................277 Chapter 20. Subshells.....................................................................................................................................278 Chapter 21. Restricted Shells .........................................................................................................................282 Chapter 22. Process Substitution ...................................................................................................................283 Chapter 23. Functions....................................................................................................................................283 Chapter 24. Aliases.........................................................................................................................................283 Chapter 25. List Constructs...........................................................................................................................283 Chapter 26. Arrays.........................................................................................................................................283 Chapter 27. Files.............................................................................................................................................284 Chapter 28. /dev and /proc.............................................................................................................................287 Chapter 29. Of Zeros and Nulls .....................................................................................................................305 Chapter 30. Debugging...................................................................................................................................306 Chapter 31. Options ........................................................................................................................................308 Chapter 32. Gotchas.......................................................................................................................................309 Chapter 33. Scripting With Style..................................................................................................................310 Chapter 34. Miscellany...................................................................................................................................312 Chapter 35. Bash, version 2...........................................................................................................................314 Chapter 36. Credits .........................................................................................................................................321 Chapter 37. Endnotes.....................................................................................................................................325 Advanced Bash−Scripting Guide iii Chapter 1. Why Shell Programming? A working knowledge of shell scripting is essential to everyone wishing to become reasonably adept at system administration, even if they do not anticipate ever having to actually write a script. Consider that as a Linux machine boots up, it executes the shell scripts in /etc/rc.d to restore the system configuration and set up services. A detailed understanding of these startup scripts is important for analyzing the behavior of a system, and possibly modifying it. Writing shell scripts is not hard to learn, since the scripts can be built in bite−sized sections and there is only a fairly small set of shell−specific operators and options [2] to learn. The syntax is simple and straightforward, similar to that of invoking and chaining together utilities at the command line, and there are only a few "rules" to learn. Most short scripts work right the first time, and debugging even the longer ones is straightforward. A shell script is a "quick and dirty" method of prototyping a complex application. Getting even a limited subset of the functionality to work in a shell script, even if slowly, is often a useful first stage in project development. This way, the structure of the application can be tested and played with, and the major pitfalls found before proceeding to the final coding in C, C++, Java, or Perl. Shell scripting hearkens back to the classical UNIX philosophy of breaking complex projects into simpler subtasks, of chaining together components and utilities. Many consider this a better, or at least more esthetically pleasing approach to problem solving than using one of the new generation of high powered all−in−one languages, such as Perl, which attempt to be all things to all people, but at the cost of forcing you to alter your thinking processes to fit the tool. When not to use shell scripts resource−intensive tasks, especially where speed is a factor (sorting, hashing, etc.) • procedures involving heavy−duty math operations, especially floating point arithmetic, arbitrary precision calculations, or complex numbers (use C++ or FORTRAN instead) • cross−platform portability required (use C instead) • complex applications, where structured programming is a necessity (need typechecking of variables, function prototypes, etc.) • mission−critical applications upon which you are betting the ranch, or the future of the company • situations where security is important, where you need to protect against hacking • project consists of subcomponents with interlocking dependencies • extensive file operations required (Bash is limited to serial file access, and that only in a particularly clumsy and inefficient line−by−line fashion) • need multi−dimensional arrays • need data structures, such as linked lists or trees • need to generate or manipulate graphics or GUIs • need direct access to system hardware • need port or socket I/O • need to use libraries or interface with legacy code • proprietary, closed−source applications (shell scripts are necessarily Open Source) • If any of the above applies, consider a more powerful scripting language, perhaps Perl, Tcl, Python, or possibly a high−level compiled language such as C, C++, or Java. Even then, prototyping the application as a shell script might still be a useful development step. Chapter 1. Why Shell Programming? 1 We will be using Bash, an acronym for "Bourne−Again Shell" and a pun on Stephen Bourne's now classic Bourne Shell. Bash has become a de facto standard for shell scripting on all flavors of UNIX. Most of the principles dealt with in this document apply equally well to scripting with other shells, such as the Korn Shell, from which Bash derives some of its features, [3] and the C Shell and its variants. (Note that C Shell programming is not recommended due to certain inherent problems, as pointed out in a news group posting by Tom Christiansen in October of 1993). The following is a tutorial in shell scripting. It relies heavily on examples to illustrate features of the shell. As far as possible, the example scripts have been tested, and some of them may actually be useful in real life. The reader should use the actual examples in the the source archive (something−or−other.sh), [4] give them execute permission (chmod u+rx scriptname), then run them to see what happens. Should the source archive not be available, then cut−and−paste from the HTML, pdf, or text rendered versions. Be aware that some of the scripts below introduce features before they are explained, and this may require the reader to temporarily skip ahead for enlightenment. Unless otherwise noted, the author of this document wrote the example uploads/Management/ abs-guide 3 .pdf
Documents similaires
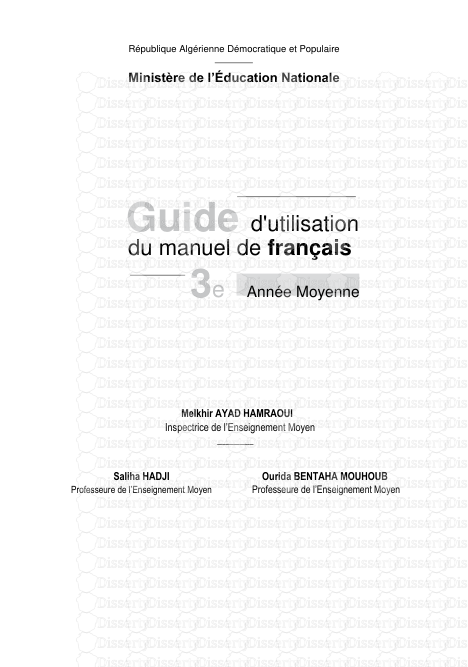
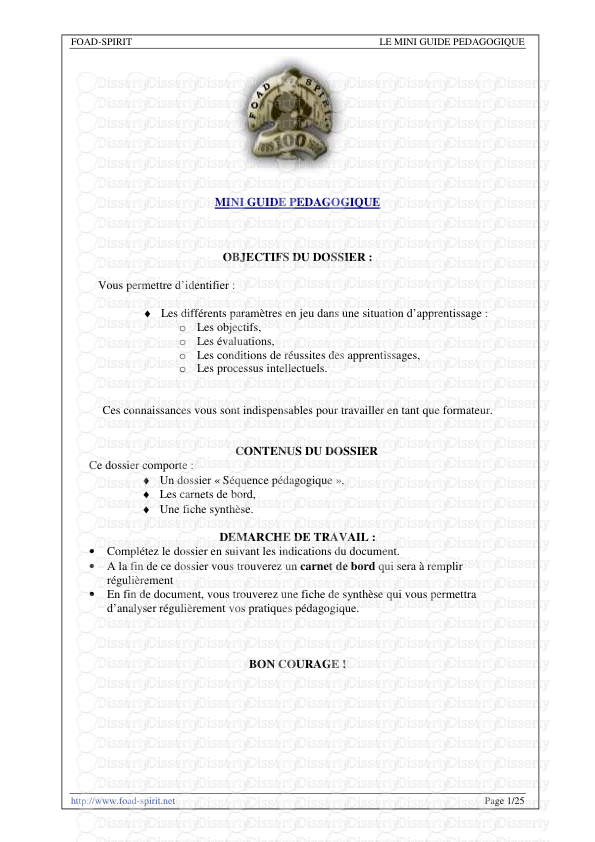
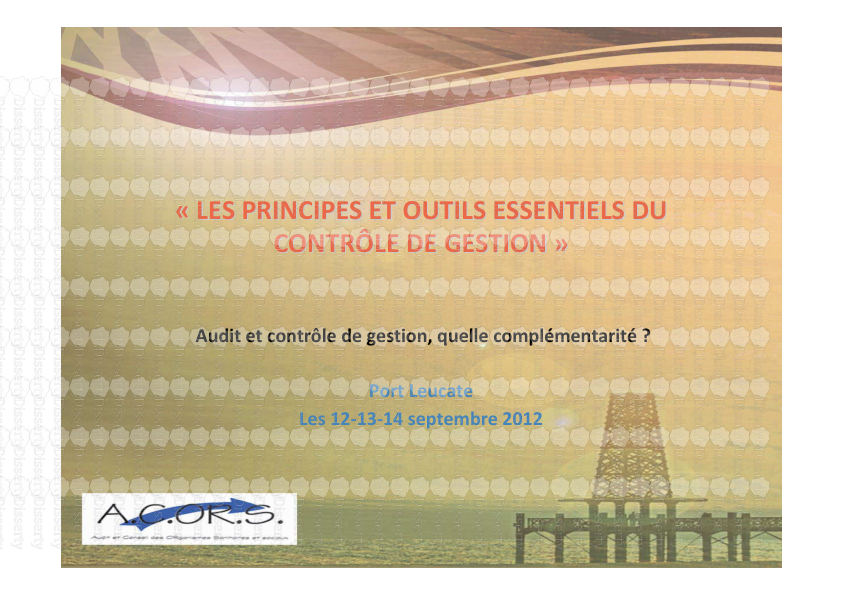
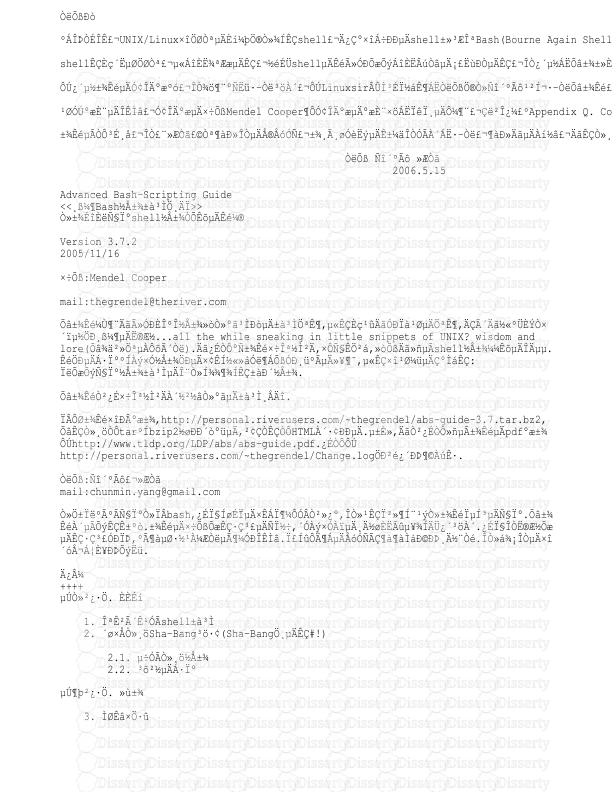
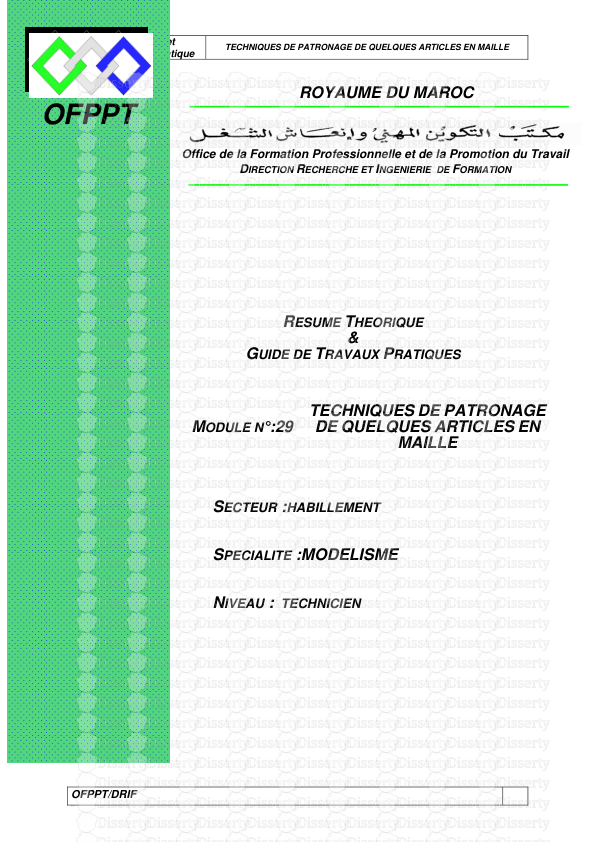
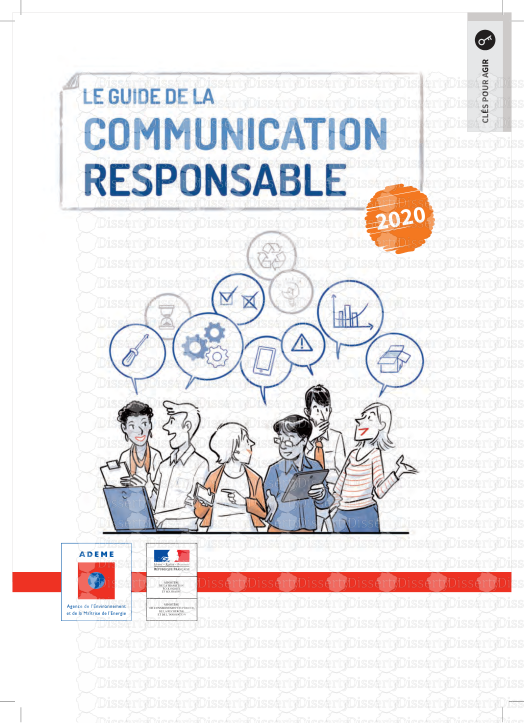
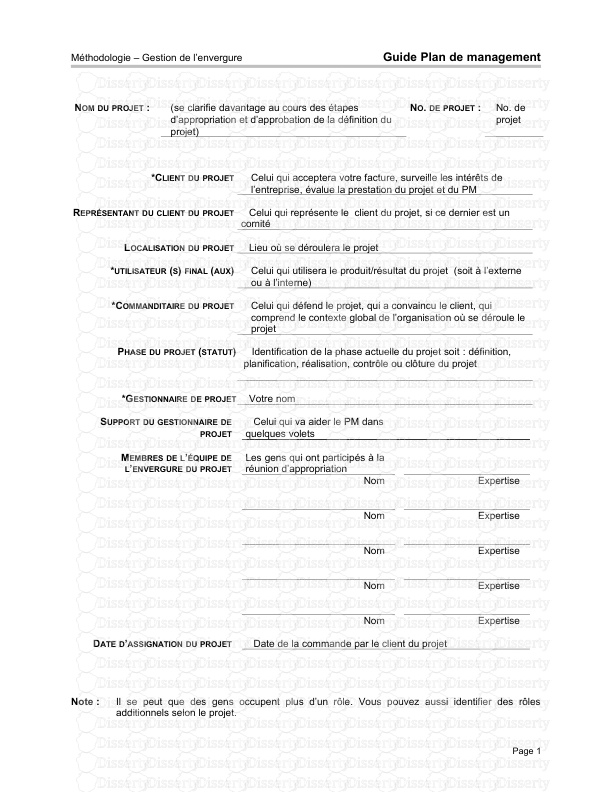

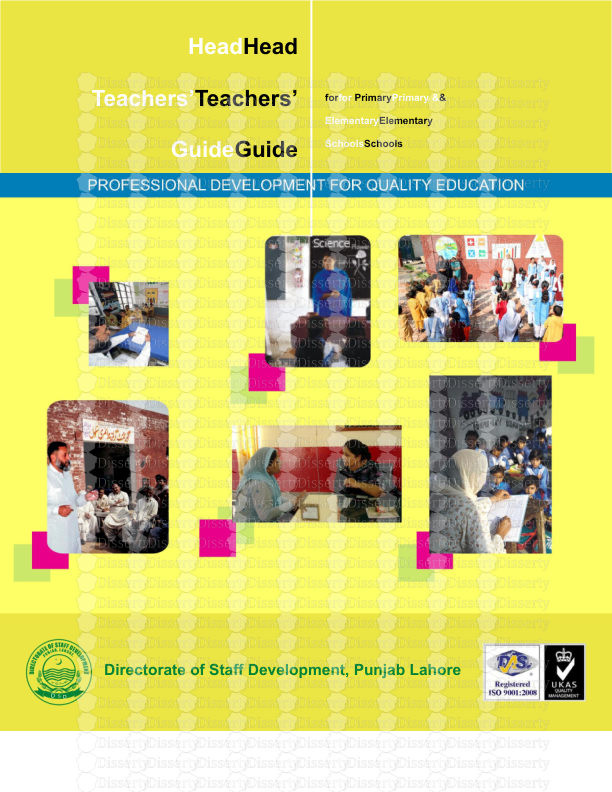
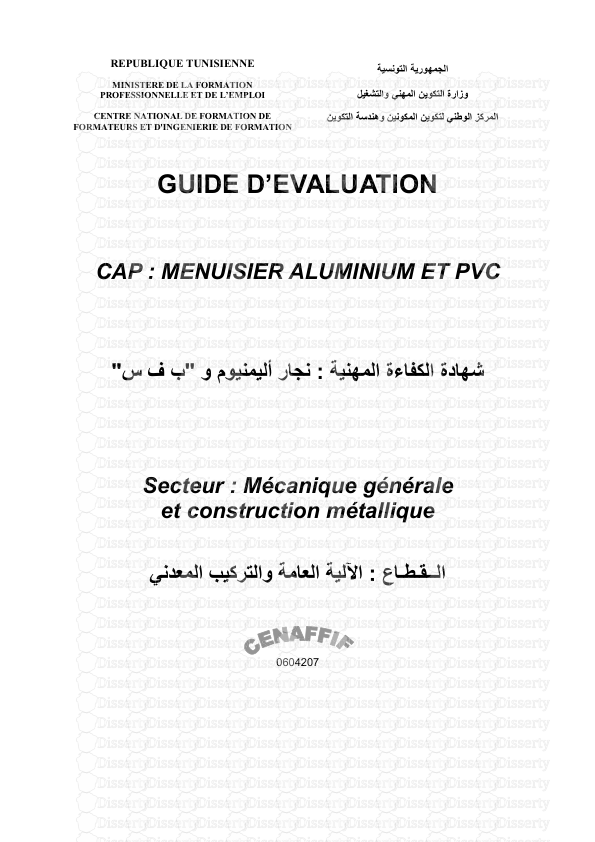
-
23
-
0
-
0
Licence et utilisation
Gratuit pour un usage personnel Attribution requise- Détails
- Publié le Fev 07, 2022
- Catégorie Management
- Langue French
- Taille du fichier 0.7746MB